Hey my tech family, Let’s learn to create a loan calculator using HTML and JavaScript with source code. In this loan calculator, if we enter the amount, Percentage, and number of years, it shows years then it is going to display the total payment, monthly payment, and total interest.
Read also : 15+ Calculators Template Using JavaScript (Demo + Code)
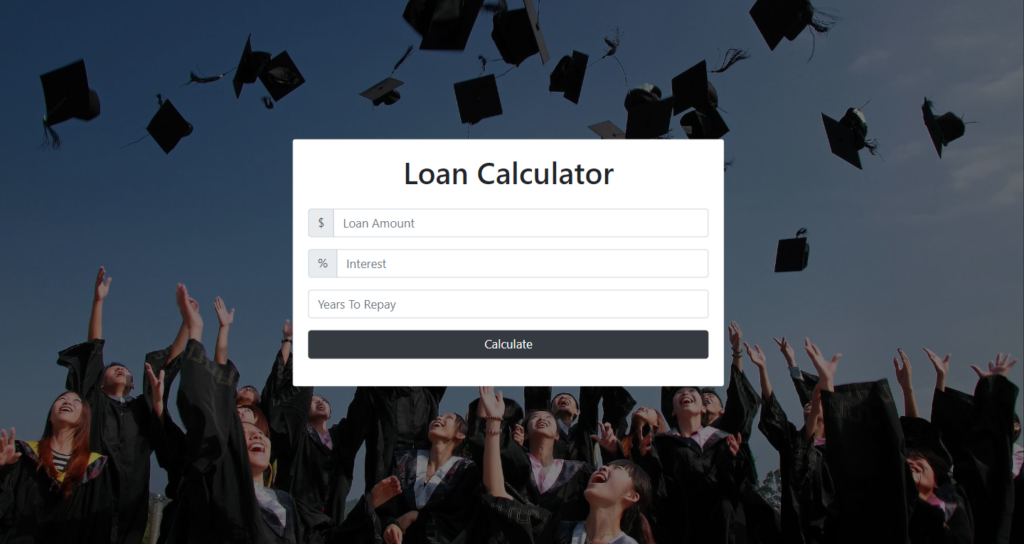
What is the benefit of creating a Loan Calculator?
Nowadays people always want a customized or engaging interface along with great functionality, in certain situations creating a loan calculator could be very helpful, it could be also called an EMI calculator.
So without any delay Let us get started with the source code.
Here is the EMI calculator that we are gonna make !!!

First, let’s see the HTML CODE :
<!doctype html>
<html lang="en">
<head>
<!-- Required meta tags -->
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<!-- Bootstrap CSS -->
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.4.1/css/bootstrap.min.css" integrity="sha384-Vkoo8x4CGsO3+Hhxv8T/Q5PaXtkKtu6ug5TOeNV6gBiFeWPGFN9MuhOf23Q9Ifjh" crossorigin="anonymous">
<style>
#loading, #results{
display: none;
}
</style>
<title>Loan Calculator</title>
</head>
<body class="bg-dark">
<div class="container">
<div class="row">
<div class="col-md-6 mx-auro">
<div class="card card-body text-center mt-5">
<h1 class="heading display-5 pb-3">Loan Calculator</h1>
<form action="" id="loan-form">
<div class="form-group">
<div class="input-group">
<span class="input-group-text">$</span>
<input type="number" class="form-control" id="amount" placeholder="Loan Amout">
</div>
</div>
<div class="form-group">
<div class="input-group">
<span class="input-group-text">%</span>
<input type="number" class="form-control" id="interest" placeholder="Interest">
</div>
</div>
<div class="form-group">
<input type="number" class="form-control" id="years" placeholder="Years to repay">
</div>
<div class="form-group">
<input type="submit" value="Calculator" class="btn btn-dark btn-block">
</div>
</form>
<!-- Loader Here -->
<div id="loading">
<img src="img/loading.gif" alt="">
</div>
<!-- Results -->
<div id="results" class="pt-4">
<h5>Results</h5>
<div class="form-group">
<div class="input-group">
<span class="input-group-text">Total Payment</span>
<input type="number" class="form-control" id="total-payment" disabled>
</div>
</div>
<div class="form-group">
<div class="input-group">
<span class="input-group-text">Mothly Payment</span>
<input type="number" class="form-control" id="monthly-payment" disabled>
</div>
</div>
<div class="form-group">
<div class="input-group">
<span class="input-group-text">Total Interest</span>
<input type="number" class="form-control" id="total-interest" disabled>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
<!-- Optional JavaScript -->
<!-- jQuery first, then Popper.js, then Bootstrap JS -->
<script src="https://code.jquery.com/jquery-3.4.1.slim.min.js" integrity="sha384-J6qa4849blE2+poT4WnyKhv5vZF5SrPo0iEjwBvKU7imGFAV0wwj1yYfoRSJoZ+n" crossorigin="anonymous"></script>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/umd/popper.min.js" integrity="sha384-Q6E9RHvbIyZFJoft+2mJbHaEWldlvI9IOYy5n3zV9zzTtmI3UksdQRVvoxMfooAo" crossorigin="anonymous"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.4.1/js/bootstrap.min.js" integrity="sha384-wfSDF2E50Y2D1uUdj0O3uMBJnjuUD4Ih7YwaYd1iqfktj0Uod8GCExl3Og8ifwB6" crossorigin="anonymous"></script>
<script src="app.js"></script>
</body>
</html>
Now we will see the javascript code :
// //Listen for submit button //document.getElementById('loan-form').addEventListener('submit',calculateResults);; //Listen for submit button const form = document.getElementById('loan-form'); //form.addEventListener('submit',calculateResults);//Without loader form.addEventListener('submit',function(e){ //Hide Results document.getElementById('results').style.display='none'; //Show Loader document.getElementById('loading').style.display='block'; setTimeout(calculateResults,2000); e.preventDefault(); }); //Calculate Results function function calculateResults(e){ console.log('calculating...'); //UI cars const ELamount = document.getElementById('amount'); const ELinterest = document.getElementById('interest'); const ELyears = document.getElementById('years'); const ELMonthly_payment = document.getElementById('monthly-payment'); const ELtotal_payment = document.getElementById('total-payment'); const ELtotal_interest = document.getElementById('total-interest'); const principal = parseFloat(ELamount.value); const calculatedInterest = parseFloat(ELinterest.value) /100 /12; const calculatedPayment = parseFloat(ELyears.value )*12; //Create monthly payment const x = Math.pow(1 + calculatedInterest, calculatedPayment); const monthly = (principal * x * calculatedInterest) / (x - 1); console.log(monthly); if(isFinite(monthly)){//check whether it is finite or not ELMonthly_payment.value = monthly.toFixed(2); ELtotal_payment.value = (monthly*calculatedPayment).toFixed(2); ELtotal_interest.value = ((monthly*calculatedPayment)-principal).toFixed(2);//fix to 2 deciman places //Show Results document.getElementById('results').style.display='block'; //Hide Loader document.getElementById('loading').style.display='none'; }else{ console.log("Plase check your numbers"); //Display an error showError('Plase check your number'); } e.preventDefault(); } function showError(error){ //Show Results document.getElementById('results').style.display='none'; //Hide Loader document.getElementById('loading').style.display='none'; //-------------------------------------------------------------------- //Create a div const errorDiv = document.createElement('div'); //get elements const ELcard = document.querySelector ('.card'); const ELheading = document.querySelector('.heading'); //Add class errorDiv.className = 'alert alert-danger'; //create text node and append to dic errorDiv.appendChild(document.createTextNode(error)); //Insert error above heading ELcard.insertBefore(errorDiv, ELheading); //clear error after 3 seconds setTimeout(clearError, 3000); } function clearError(){ document.querySelector('.alert').remove(); }
Output after applying both HTML and Javascript:
Final Output of Loan Calculator Using JavaScript Source Code
Read also: 50+HTML, CSS and JavaScript Projects With Source Code
Now we have completed our Loan Calculator. Here is our updated output with HTML and JavaScript. Hope you like the Loan Calculator Project. you can see the output video and project screenshots. See our other blogs and gain knowledge in front-end development.
Thank you!
In this post, we learned how to create a Loan Calculator Using HTML and JavaScript Source Code. For queries, please drop a comment to reply or help you in easy learning.
Written by – Code With Random/Anki
FAQ related to Loan calculator
is this project responsive or not?
Yes! This is a responsive project
Do you use any external links to create this project?
Yes!
What is the use of making a loan/EMI calculator?
Nowadays people always want a customized or engaging interface along with great functionality, in certain situations creating a loan calculator could be very helpful, it could be also called an EMI calculator.