Restaurant Menu System using C++ (With Source Code)
Hello, coders. Welcome to the codewithrandom blog. In this article, we will learn how to create a Restaurant Menu System using C++ (With Source Code).
A Restaurant Menu System using C++ programming language is a basic implantation of a menu system. The program allows the user to choose an order and the number of offers they want, then calculates the total price depending on the order and the number of deals and shows the order information.
The program starts by showing the menu selections, which include the names and prices of various food items. The user is asked to input their order number as well as the quantity of discounts they want.
Rock Paper and Scissors Game using C++ (With Source Code)
Features of the system:
- It presents a menu to the user.
- Allows user to select an item and specify the number of deals they want.
- The program then calculates the total price based on the order and the number of deals and displays the order details.
Overview of the UI:
- Suppose user selects option 1:
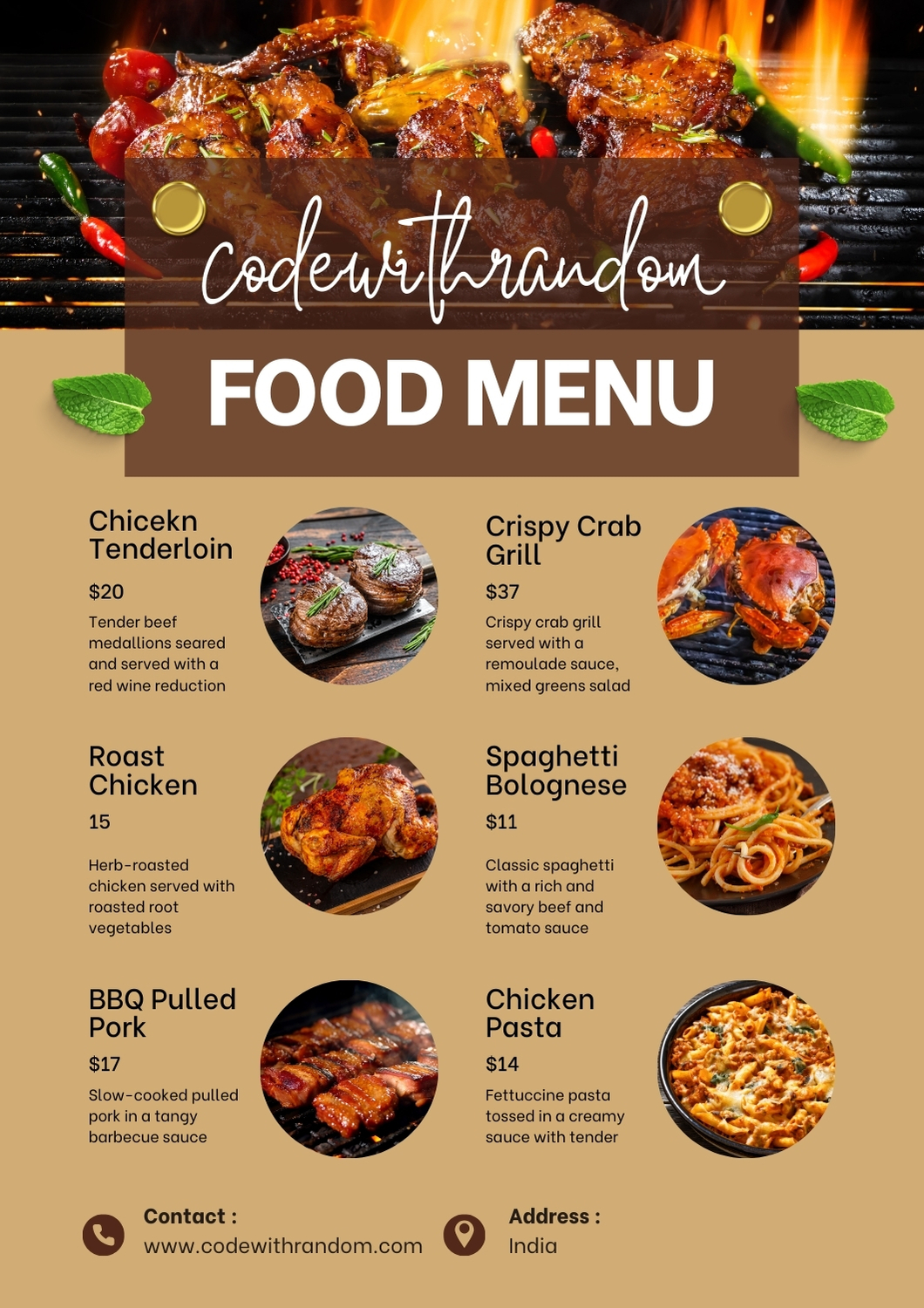
Source code:
You can directly use this code by copying it in your IDE to understand the working and then can create it by understanding the project.
//restaurant menu system #include <iostream> using namespace std; int main(){ int order,no_deals; cout<<"|*---------------------------MENU-----------------------------*|"<<endl; cout<<endl; cout<<"(1) Chicken Biryani $2 only."<<endl; cout<<"(2) Chicken Palao $1.5 only."<<endl; cout<<"(3) Chinese Rice $2.5 only."<<endl; cout<<"(4) Chicken Burgar $1 only."<<endl; cout<<"(5) Nawabi Pizza $4.5 only."<<endl; cout<<"(6) 2.5 Litre Coke $1.75 only."<<endl; cout<<endl; cout<<"Please select the order number: "; cin>>order; cout<<"Please enter the number of deals: "; cin>>no_deals; cout<<endl; switch(order) { case 1: cout<<"Order : Chicken Biryani."<<endl; cout<<"Number of deals : "<<no_deals<<endl; cout<<"Price of each deal: $2 only."<<endl; cout<<"Total price : "<<"$"<<2*no_deals<<" "<<"only."<<endl; cout<<endl; cout<<"~---------THANK YOU FOR COMING-----------~"<<endl; break; case 2: cout<<"Order Chicken Palao."<<endl; cout<<"Number of deals : "<<no_deals<<endl; cout<<"Price of each deal: $1.5 only."<<endl; cout<<"Total price : "<<"$"<<1.5*no_deals<<" "<<"only."<<endl; cout<<endl; cout<<"~---------THANK YOU FOR COMING-----------~"<<endl; break; case 3: cout<<"Order : Chinese Rice."<<endl; cout<<"Number of deals : "<<no_deals<<endl; cout<<"Price of each deal: $2.5 only."<<endl; cout<<"Total price : "<<"$"<<2.5*no_deals<<" "<<"only."<<endl; cout<<endl; cout<<"~---------THANK YOU FOR COMING-----------~"<<endl; break; case 4: cout<<"Order : Chicken Burgar."<<endl; cout<<"Number of deals : "<<no_deals<<endl; cout<<"Price of each deal: $1 only."<<endl; cout<<"Total price : "<<"$"<<1*no_deals<<" "<<"only."<<endl; cout<<endl; cout<<"~---------THANK YOU FOR COMING-----------~"<<endl; break; case 5: cout<<"Order : Nawabi Pizza ."<<endl; cout<<"Number of deals : "<<no_deals<<endl; cout<<"Price of each deal: $4.5 only."<<endl; cout<<"Total price : "<<"$"<<4.5*no_deals<<" "<<"only."<<endl; cout<<endl; cout<<"~---------THANK YOU FOR COMING-----------~"<<endl; break; case 6: cout<<"Order : 2.5 Litre Coke."<<endl; cout<<"Number of deals : "<<no_deals<<endl; cout<<"Price of each deal: $1.75 only."<<endl; cout<<"Total price : "<<"$"<<1.75*no_deals<<" "<<"only."<<endl; cout<<endl; cout<<"~---------THANK YOU FOR COMING-----------~"<<endl; break; } return 0; }
Now let us understand the code:-
- We will start by writing the header of the code with the required libraries – iostream (input and output stream). We will write “using namespace std;” line to avoid having to write “std::” before certain standard library functions.
- Now creating the main() function where the program will begin it’s execution. We will create 2 variables – order and no-deals.
- We will print out the menu options available in the restaurant menu. Along with the menu items of food in the menu we will also display the price.
- We will write switch case statements to select between the options of menu items. Suppose a user selects option 1 – Chicken Biryani. For the details we will enter – number of deals, price of each deal and total price for the number of deals will be generated. Total 6 cases in the switch case will be created. We can add more items in the menu according to the the customization of user.
- The break statement is used to exit the switch statement.
- Similarly we will create cases for the other menu options as well and enter details upon option selection. A thankyou message will appear at the end of cost calculation.
- Finally, the main function returns 0 to indicate successful execution of the program.
- This sums up our project of Restaurant Menu System using C++.
- We will prompt the user to select the order number and the number of details. The user will enter the details using input stream.
Shop Billing System using C++ (With Source Code)
final Output:-
Here is an example to show how this project works.
Video Output:
Conclusion
We have reached the end of this article and have a lot more projects in C++ coming so stay tuned. We have started with awesome and fun projects for you all to understand C++. Learning C++ by creating fun projects makes learning easy and interesting.
Simple Interest Calculator using C++ (With Source Code)
If you enjoyed the article and learned something new today, let us know in the comments.
Thank you.
Happy Reading! 🙂
Follow: CodewithRandom
Stay With Us!