Hello Coder! Welcome to Codewithrandom Blog. Are you planning to create a Quiz App using HTML, CSS and JavaScript ? In this article, We create a Quiz App using HTML, CSS, And JavaScript. In this Project, we Score System and Previous and Next Question and After Quiz Done we can check right answer.
Quiz App Using HTML CSS and JavaScript
The main purpose of making a Quiz app in HTML CSS JavaScript is to make javascript concepts clearer and give users access to a web app so they may practice for competitive exams and improve their general knowledge. We’ll build a quiz app in this article.
Before we dive into the step-by-step process of creating a quiz app, let’s understand some of the general concepts of JavaScript for creating quiz apps.
JavaScript Projects With Source Code
Video Tutorial Of Quiz App Using JavaScript
I hope you enjoy this video tutorial so let’s start with a basic HTML structure for the Quiz App Using JavaScript.
Quiz HTML Code:-
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Javascript Quiz</title> <link rel="stylesheet" href="style1.css"> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.css"> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.0.0-alpha.6/css/bootstrap.min.css"> </head> <body> <header class="header bg-primary"> <div class="left-title">JS Quiz</div> <div class="right-title">Total Questions: <span id="tque"></span></div> <div class="clearfix"></div> </header> <div class="content"> <div class="container-fluid"> <div class="row"> <div class="col-sm-12"> <div id="result" class="quiz-body"> <form name="quizForm" onSubmit=""> <fieldset class="form-group"> <h4><span id="qid">1.</span> <span id="question"></span></h4> <div class="option-block-container" id="question-options"> </div> <!-- End of option block --> </fieldset> <button name="previous" id="previous" class="btn btn-success">Previous</button> <button name="next" id="next" class="btn btn-success">Next</button> </form> </div> </div> <!-- End of col-sm-12 --> </div> <!-- End of row --> </div> <!-- ENd of container fluid --> </div> <!-- End of content --> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.1.1/jquery.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.0.0-alpha.6/js/bootstrap.min.js"></script> <script src="app.js"></script> </body> </html>
HTML Structure :
<link rel="stylesheet" href="style1.css"> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.css"> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.0.0-alpha.6/css/bootstrap.min.css">
Before continuing, If you want to learn complete frontend web development then we have launched an E-Book “Master Frontend Development: Zero to Hero” for you. This E-Book includes hand-crafted lessons on HTML, CSS, JavaScript, and Bootstrap. And also 100+ frontend projects and interview questions as well.
HTML output for quiz app:-
CSS Code For Quiz App
.content { margin-top: 54px; } .header { padding: 15px; position: fixed; top: 0; width: 100%; z-index: 9999; } .left-title { width: 80px; color: #fff; font-size: 18px; float: left; } .right-title { width: 150px; text-align: right; float: right; color: #fff; } .quiz-body { margin-top: 15px; padding-bottom: 50px; } .option-block-container { margin-top: 20px; max-width: 420px; } .option-block { padding: 10px; background: aliceblue; border: 1px solid #84c5fe; margin-bottom: 10px; cursor: pointer; } .result-question { font-weight: bold; } .c-wrong { margin-left: 20px; color: #ff0000; } .c-correct { margin-left: 20px; color: green; } .last-row { border-bottom: 1px solid #ccc; padding-bottom: 25px; margin-bottom: 25px; } .res-header { border-bottom: 1px solid #ccc; margin-bottom: 15px; padding-bottom: 15px; }
Create Scroll Arrow Animation Using HTML and CSS
Updated output With HTML and CSS code For the Quiz App
JavaScript Code For Quiz App:-
/* Created and coded by Abhilash Narayan */ /* Quiz source: w3schools.com */ var quiz = { "JS": [ { "id": 1, "question": "Inside which HTML element do we put the JavaScript?", "options": [ { "a": "<script>", "b": "<javascript>", "c": "<scripting>", "d": "<js>" } ], "answer": "<script>", "score": 0, "status": "" }, { "id": 2, "question": "Where is the correct place to insert a JavaScript?", "options": [ { "a": "The <head> section", "b": "The <body> section", "c": "Both the <head> section and the <body> section are correct" } ], "answer": "Both the <head> section and the <body> section are correct", "score": 0, "status": "" }, { "id": 3, "question": "What is the correct syntax for referring to an external script called 'xxx.js'?", "options": [ { "a": "<script href="xxx.js">", "b": "<script name="xxx.js">", "c": "<script src="xxx.js">" } ], "answer": "<script src="xxx.js">", "score": 0, "status": "" }, { "id": 4, "question": "The external JavaScript file must contain the <script> tag.", "options": [ { "a": "True", "b": "False" } ], "answer": "False", "score": 0, "status": "" }, { "id": 5, "question": "How do you write "Hello World" in an alert box?", "options": [ { "a": "alertBox("Hello World");", "b": "msg("Hello World");", "c": "alert("Hello World");", "d": "msgBox("Hello World");", } ], "answer": "alert("Hello World");", "score": 0, "status": "" }, { "id": 6, "question": "How do you create a function in JavaScript?", "options": [ { "a": "function myFunction()", "b": "function:myFunction()", "c": "function = myFunction()", } ], "answer": "function myFunction()", "score": 0, "status": "" }, { "id": 7, "question": "How do you call a function named "myFunction"?", "options": [ { "a": "call function myFunction()", "b": "call myFunction()", "c": "myFunction()", } ], "answer": "myFunction()", "score": 0, "status": "" }, { "id": 8, "question": "How to write an IF statement in JavaScript?", "options": [ { "a": "if i = 5 then", "b": "if i == 5 then", "c": "if (i == 5)", "d": " if i = 5", } ], "answer": "if (i == 5)", "score": 0, "status": "" }, { "id": 9, "question": "Which of the following is a disadvantage of using JavaScript?", "options": [ { "a": "Client-side JavaScript does not allow the reading or writing of files.", "b": "JavaScript can not be used for Networking applications because there is no such support available.", "c": "JavaScript doesn't have any multithreading or multiprocess capabilities.", "d": "All of the above." } ], "answer": "All of the above.", "score": 0, "status": "" }, { "id": 10, "question": "How to write an IF statement for executing some code if "i" is NOT equal to 5?", "options": [ { "a": "if (i <> 5)", "b": "if i <> 5", "c": "if (i != 5)", "d": "if i =! 5 then", } ], "answer": "if (i != 5)", "score": 0, "status": "" }, { "id": 11, "question": "How does a WHILE loop start?", "options": [ { "a": "while i = 1 to 10", "b": "while (i <= 10; i++)", "c": "while (i <= 10)" } ], "answer": "while (i <= 10)", "score": 0, "status": "" }, { "id": 12, "question": "How does a FOR loop start?", "options": [ { "a": "for (i = 0; i <= 5)", "b": "for (i = 0; i <= 5; i++)", "c": "for i = 1 to 5", "d": "for (i <= 5; i++)" } ], "answer": "for (i = 0; i <= 5; i++)", "score": 0, "status": "" }, { "id": 13, "question": "How can you add a comment in a JavaScript?", "options": [ { "a": "//This is a comment", "b": "‚This is a comment", "c": "<!--This is a comment-->" } ], "answer": "//This is a comment", "score": 0, "status": "" }, { "id": 14, "question": "How to insert a comment that has more than one line?", "options": [ { "a": "/*This comment has more than one line*/", "b": "//This comment has more than one line//", "c": "<!--This comment has more than one line-->" } ], "answer": "/*This comment has more than one line*/", "score": 0, "status": "" }, { "id": 15, "question": "What is the correct way to write a JavaScript array?", "options": [ { "a": "var colors = (1:"red", 2:"green", 3:"blue")", "b": "var colors = ["red", "green", "blue"]", "c": "var colors = 1 = ("red"), 2 = ("green"), 3 = ("blue")", "d": "var colors = "red", "green", "blue"" } ], "answer": "var colors = ["red", "green", "blue"]", "score": 0, "status": "" }, { "id": 16, "question": "How do you round the number 7.25, to the nearest integer?", "options": [ { "a": "rnd(7.25)", "b": "Math.round(7.25)", "c": "Math.rnd(7.25)", "d": "round(7.25)" } ], "answer": "Math.round(7.25)", "score": 0, "status": "" }, { "id": 17, "question": "How do you find the number with the highest value of x and y?", "options": [ { "a": "Math.max(x, y)", "b": "Math.ceil(x, y)", "c": "top(x, y)", "d": "ceil(x, y)" } ], "answer": "Math.max(x, y)", "score": 0, "status": "" }, { "id": 18, "question": "What is the correct JavaScript syntax for opening a new window called "w2"?", "options": [ { "a": "w2 = window.new("http://www.w3schools.com");", "b": "w2 = window.open("http://www.w3schools.com");" } ], "answer": "w2 = window.open("http://www.w3schools.com");", "score": 0, "status": "" }, { "id": 19, "question": "JavaScript is the same as Java.", "options": [ { "a": "true", "b": "false" } ], "answer": "false", "score": 0, "status": "" }, { "id": 20, "question": "How can you detect the client’s browser name?", "options": [ { "a": "navigator.appName", "b": "browser.name", "c": "client.navName" } ], "answer": "navigator.appName", "score": 0, "status": "" }, { "id": 21, "question": "Which event occurs when the user clicks on an HTML element?", "options": [ { "a": "onchange", "b": "onclick", "c": "onmouseclick", "d": "onmouseover" } ], "answer": "onclick", "score": 0, "status": "" }, { "id": 22, "question": "How do you declare a JavaScript variable?", "options": [ { "a": "var carName;", "b": "variable carName;", "c": "v carName;" } ], "answer": "var carName;", "score": 0, "status": "" }, { "id": 23, "question": "Which operator is used to assign a value to a variable?", "options": [ { "a": "*", "b": "-", "c": "=", "d": "x" } ], "answer": "=", "score": 0, "status": "" }, { "id": 24, "question": "What will the following code return: Boolean(10 > 9)", "options": [ { "a": "NaN", "b": "false", "c": "true" } ], "answer": "true", "score": 0, "status": "" }, { "id": 25, "question": "Is JavaScript case-sensitive?", "options": [ { "a": "No", "b": "Yes" } ], "answer": "Yes", "score": 0, "status": "" } ] } var quizApp = function () { this.score = 0; this.qno = 1; this.currentque = 0; var totalque = quiz.JS.length; this.displayQuiz = function (cque) { this.currentque = cque; if (this.currentque < totalque) { $("#tque").html(totalque); $("#previous").attr("disabled", false); $("#next").attr("disabled", false); $("#qid").html(quiz.JS[this.currentque].id + '.'); $("#question").html(quiz.JS[this.currentque].question); $("#question-options").html(""); for (var key in quiz.JS[this.currentque].options[0]) { if (quiz.JS[this.currentque].options[0].hasOwnProperty(key)) { $("#question-options").append( "<div class='form-check option-block'>" + "<label class='form-check-label'>" + "<input type='radio' class='form-check-input' name='option' id='q" + key + "' value='" + quiz.JS[this.currentque].options[0][key] + "'><span id='optionval'>" + quiz.JS[this.currentque].options[0][key] + "</span></label>" ); } } } if (this.currentque <= 0) { $("#previous").attr("disabled", true); } if (this.currentque >= totalque) { $('#next').attr('disabled', true); for (var i = 0; i < totalque; i++) { this.score = this.score + quiz.JS[i].score; } return this.showResult(this.score); } } this.showResult = function (scr) { $("#result").addClass('result'); $("#result").html("<h1 class='res-header'>Total Score: " + scr + '/' + totalque + "</h1>"); for (var j = 0; j < totalque; j++) { var res; if (quiz.JS[j].score == 0) { res = '<span class="wrong">' + quiz.JS[j].score + '</span><i class="fa fa-remove c-wrong"></i>'; } else { res = '<span class="correct">' + quiz.JS[j].score + '</span><i class="fa fa-check c-correct"></i>'; } $("#result").append( '<div class="result-question"><span>Q ' + quiz.JS[j].id + '</span> ' + quiz.JS[j].question + '</div>' + '<div><b>Correct answer:</b> ' + quiz.JS[j].answer + '</div>' + '<div class="last-row"><b>Score:</b> ' + res + '</div>' ); } } this.checkAnswer = function (option) { var answer = quiz.JS[this.currentque].answer; option = option.replace(/</g, "<") //for < option = option.replace(/>/g, ">") //for > option = option.replace(/"/g, """) if (option == quiz.JS[this.currentque].answer) { if (quiz.JS[this.currentque].score == "") { quiz.JS[this.currentque].score = 1; quiz.JS[this.currentque].status = "correct"; } } else { quiz.JS[this.currentque].status = "wrong"; } } this.changeQuestion = function (cque) { this.currentque = this.currentque + cque; this.displayQuiz(this.currentque); } } var jsq = new quizApp(); var selectedopt; $(document).ready(function () { jsq.displayQuiz(0); $('#question-options').on('change', 'input[type=radio][name=option]', function (e) { //var radio = $(this).find('input:radio'); $(this).prop("checked", true); selectedopt = $(this).val(); }); }); $('#next').click(function (e) { e.preventDefault(); if (selectedopt) { jsq.checkAnswer(selectedopt); } jsq.changeQuestion(1); }); $('#previous').click(function (e) { e.preventDefault(); if (selectedopt) { jsq.checkAnswer(selectedopt); } jsq.changeQuestion(-1); });
First, we built an object in our JavaScript, and then we added a question with a certain ID number to that object. We have built 25 questions in total and placed them within our object. The user will receive the quiz task’s outcome at the end of the quiz by utilizing the conditional statements to verify, as the user clicks on any of the alternatives, if and else statements, whether the answer they choose matches the proper response.
10+ HTML CSS Projects For Beginners with Source Code
Final Output For Quiz App Using JavaScript
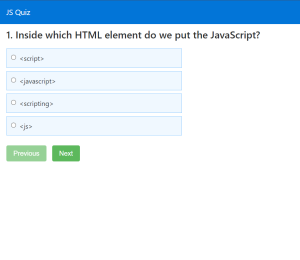
ADVERTISEMENT
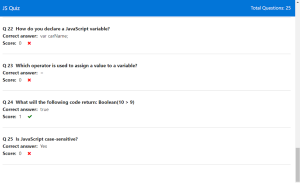
ADVERTISEMENT
Now that we have completed our JavaScript section Quiz App. our updated output with Html, CSS, and JavaScript For Quiz App.
ADVERTISEMENT
Final Video Output:
ADVERTISEMENT
ADVERTISEMENT
Hope you like the Quiz App. you can see the output video and project screenshots. See our other blogs and gain knowledge in front-end development. Thank you!
Let me know how you like this HTML CSS Quiz App. If you have any query about this project(Quiz App Using HTML CSS and JavaScript) you can comment me.
I hope you enjoyed reading this. Tell your other developers about this. You can explore our website for more such animation effects.
Thanks for visiting our website
Stay with us😊🌹
Follow on Instagram For Interesting Coding Post :- codewith_random
Thanks for posting this. Will take a closer look to increase my skill set. 👍
That’s very good one