Create Number Guessing Game Using JavaScript
Hello Coder! Welcome to the Codewithrandom blog. In this article, we create a Number Guessing Game Using JavaScript. We use If/Else Function in JavaScript Code For Show Message on Screen that guesses the number right or not.
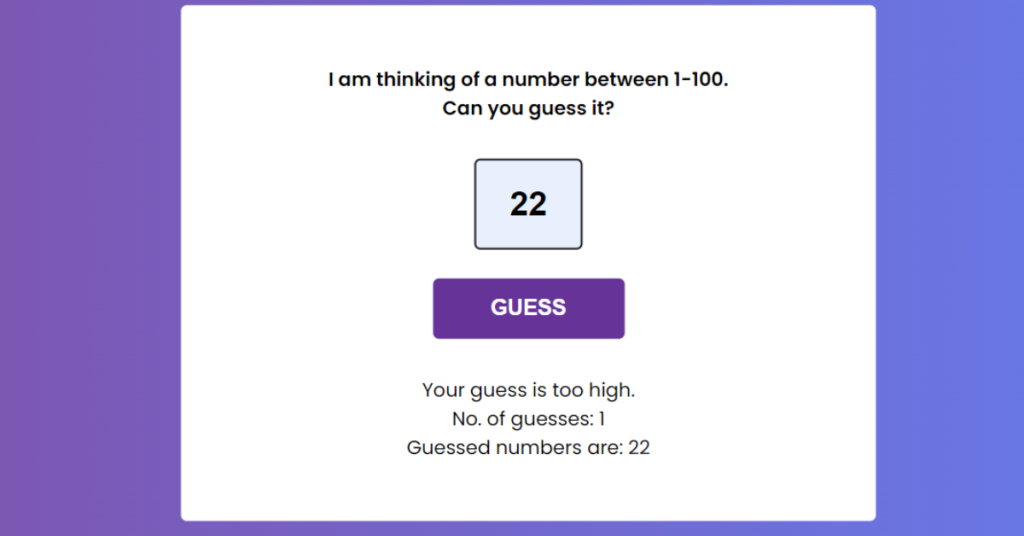
A user is required to estimate a number between 0 and 100 in a maximum of 10 attempts in a number-guessing game. If after ten tries the player cannot correctly predict the number, the game is over and he is eliminated.
50+ HTML, CSS & JavaScript Projects With Source Code
Code by | N/A |
Project Download | Link Available Below |
Language used | HTML, CSS, and JavaScript |
External link / Dependencies | NO |
Responsive | YES |
Html Code For Number Guessing Game:-
Write the html boilerplate and link it with the external Css file and Javascript File. For this project, we are using google font “Poppins” and thus add a link tag for the google fonts inside the <head> element.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta http-equiv="X-UA-Compatible" content="IE=edge" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Document</title> <link rel="stylesheet" href="style.css" /> <link rel="preconnect" href="https://fonts.gstatic.com" /> <link href="https://fonts.googleapis.com/css2?family=Poppins:wght@400;600&display=swap" rel="stylesheet" /> </head> <body> <div class="container"> <h3>I am Thinking of a number Between 1-100.</h3> <h3>Can you Guess it</h3> <input type="text" placeholder="Num" id="guess" /><br /> <button onclick="play()" id="my_btn">GUESS</button> <p id="message1">No. of Guesss : 0</p> <p id="message2">Guessed number are : none</p> <p id="message3"></p> </div> <script src="app.js"></script> </body> </html>
Portfolio Website Using HTML ,CSS ,Bootstrap and JavaScript
<link rel="stylesheet" href="style.css" /> <link rel="preconnect" href="https://fonts.gstatic.com" /> <link href="https://fonts.googleapis.com/css2?family=Poppins:wght@400;600&display=swap" rel="stylesheet" />
Adding the Structure for our number guessing game:
- Create the following div container and add the code inside the body tag for the number guessing game.
- Now inside the container, using the h3> tag, we will add a heading to the number guessing game.
- We use the input tag selector to add the field for the user to enter the number that comes to mind.
- We will now create a button when you click “play,” and we will create a “guess” button for the number guessing app.
- Then, in the end, using the paragraph tag, we will display the number of guesses and the guessed number.
<div class="container"> <h3>I am Thinking of a number Between 1-100.</h3> <h3>Can you Guess it </h3> <input type="text" placeholder="Num" id="guess"><br> <button onclick="play()" id="my_btn">GUESS</button> <p id="message1">No. of Guesss : 0</p> <p id="message2">Guessed number are : none</p> <p id="message3"></p> </div>
This HTML code provides the basic structure for the number guessing game.
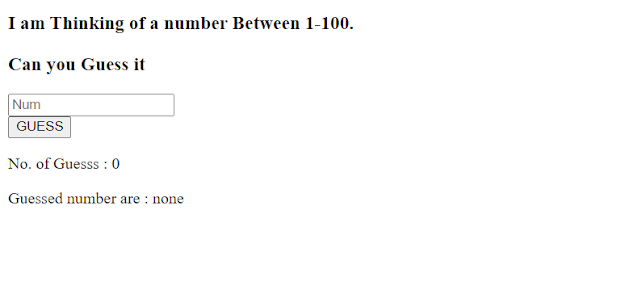
CSS Code For Number Guessing Game:-
Next, we will style our project and make it presentable using CSS. Style the background and root element to make the default padding and margin to be zero, and the box governed by the border box. Center the container element in the webpage.
Restaurant Website Using HTML and CSS
* { padding: 0; margin: 0; box-sizing: border-box; } body { height: 100vh; background: linear-gradient(to right, #7f53ac, #657ced); } .container { position: absolute; width: 50%; min-width: 580px; transform: translate(-50%, -50%); top: 50%; left: 50%; background: #fff; padding: 50px 10px; border-radius: 5px; display: grid; justify-items: center; font-family: "poppins", sans-serif; }
In order to improve the user interface, we will then style the element inside the container. The font-size and font-weight will be changed to “30px” and “600,” respectively, using the tag selector (h3).
We’ll provide “160px” for the width and “15px” for the padding using the button tag selector. We will centre the text using the text-align property, and we will use the margin-top property to establish a top margin of 30px.
Responsive Gym Website Using HTML ,CSS & JavaScript
h3 { font-size: 30px; font-weight: 600; } input[type="text"] { width: 90px; font-weight: 600; padding: 20px 0; font-size: 28px; text-align: center; margin-top: 30px; border-radius: 5px; border: 2px solid #202020; color: #663399; } button { width: 160px; padding: 15px 0; border-radius: 5px; background-color: #663399; color: #fff; border: none; font-size: 18px; font-weight: 600; margin-bottom: 30px; } p { font-weight: 400; }
Here is our updated output after css for the number guessing game.
Html Css number guessing game Output
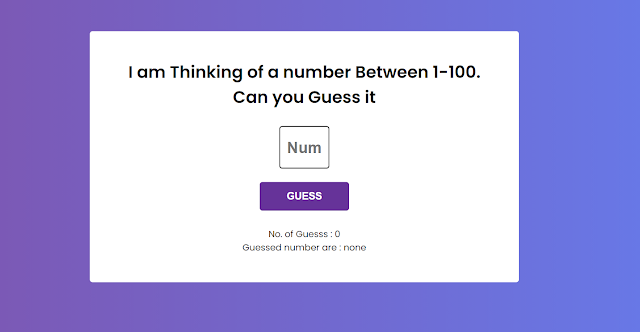
Next, we are adding functionality to our number guessing game Through Javascript.
Number Guessing Game Javascript Code:-
var msg1 = document.getElementById("message1") var msg2 = document.getElementById("message2") var msg3 = document.getElementById("message3") var answer = Math.floor(Math.random() * 100) + 1; var no_of_guesses = 0; var guesses_num = []; function play() { var user_guess = document.getElementById("guess").value; if (user_guess < 1 || user_guess > 100) { alert("Please Enter a number Between 1 to 100"); } else { guesses_num.push(user_guess); no_of_guesses += 1; if (user_guess < answer) { msg1.textContent = "Your Guess is Too low" msg2.textContent = "No. Of Guesses : " + no_of_guesses; msg3.textContent = "Guessed Number Are: " + guesses_num; } else if (user_guess > answer) { msg1.textContent = "Your Guess is Too High" msg2.textContent = "No. Of Guesses : " + no_of_guesses; msg3.textContent = "Guessed Number Are: " + guesses_num; } else if (user_guess == answer) { msg1.textContent = "Yahhhh You won It!!" msg2.textContent = "the Number was " + answer msg3.textContent = " You guessd it in " + no_of_guesses + "Guesses"; } } }
This variable’s value is predetermined to be a random number between 1 and 100. A function named play is declared in the following line (). This feature will be applied to the actual game. The text box with the id “guess” is where the user’s initial guess is obtained.
100+ JavaScript Projects With Source Code ( Beginners to Advanced)
The user is alerted and asked to submit a number between 1 and 100 if their prediction is less than 1 or greater than 100.
If not, it increments the array’s index and adds their guess to the “guesses num” array in order to keep track of how many guesses have been made so far.
If they were unlucky enough to guess correctly, the message that they got it right is displayed on msg3, along with how many guesses were made before guessing correctly and what was guessed in total numbers. If they guessed too low (less than the answer), the message that they got it wrong is displayed on msg1; if they guessed too high (more than the answer), the message that they got it right is displayed on msg2.
Now we have completed our javascript section for the number guessing game. Here is our updated output with html, css, and javascript for the number guessing game.
[su_button id=”download” url=”https://drive.google.com/drive/folders/1qjkT4UNT_wFq55xArEcrGG7T4Z1_BNpB?usp=sharing” target=”blank” style=”3d” size=”11″ wide=”yes” center=”yes” icon=”icon: download”]DOWNLOAD CODE NOW[/su_button]
You can see the output video and project screenshots of the number guessing game.
Final Output Of Number Guessing Game Javascript:-
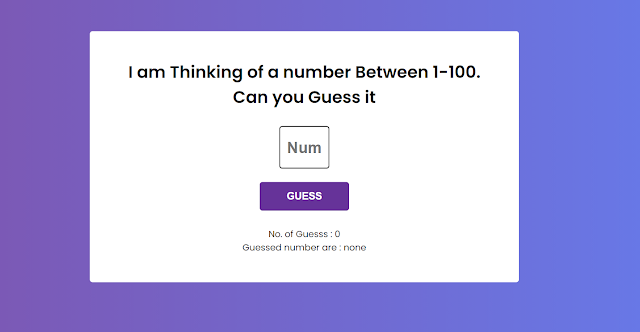
Portfolio Website Using HTML CSS And JAVASCRIPT ( Source Code)
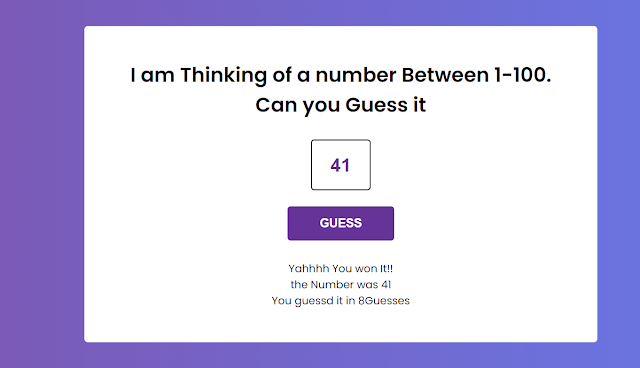
5+ HTML CSS Projects With Source Code
Video Output Of Guess The Number Game In Javascript:
Conclusion
Hope you like the Number Guessing Game Using Javascript.In this post, we learned how to create a number guessing game using simple HTML & CSS, and javascript. If we made a mistake or for any confusion, please drop a comment to help our readers and make the process easy for all of us.
ADVERTISEMENT
See our other blogs and gain knowledge in front-end development. Thank you
ADVERTISEMENT
Written by – Code With Random/Anki
ADVERTISEMENT
Which code editor do you use for this Number Guessing Game coding?
I personally recommend using VS Code Studio, it’s straightforward and easy to use.
ADVERTISEMENT
is this project responsive or not?
Yes! this project is a responsive project.
ADVERTISEMENT
Do you use any external links to create this project?
No!