BMI Calculator using HTML and JavaScript (Source Code)
Hello coders👨💻!! In this article will learn how to make a BMI Calculator out of HTML, CSS, and JavaScript. Body Mass Index (BMI) is an abbreviation for Body Mass Index. It essentially aids in determining how much mass we have in our bodies.
A healthy and normal BMI should be between 18.5 and 25. It is underweight if its value is less than 18, which is unhealthy. It is considered fat or overweight if its value exceeds 25.
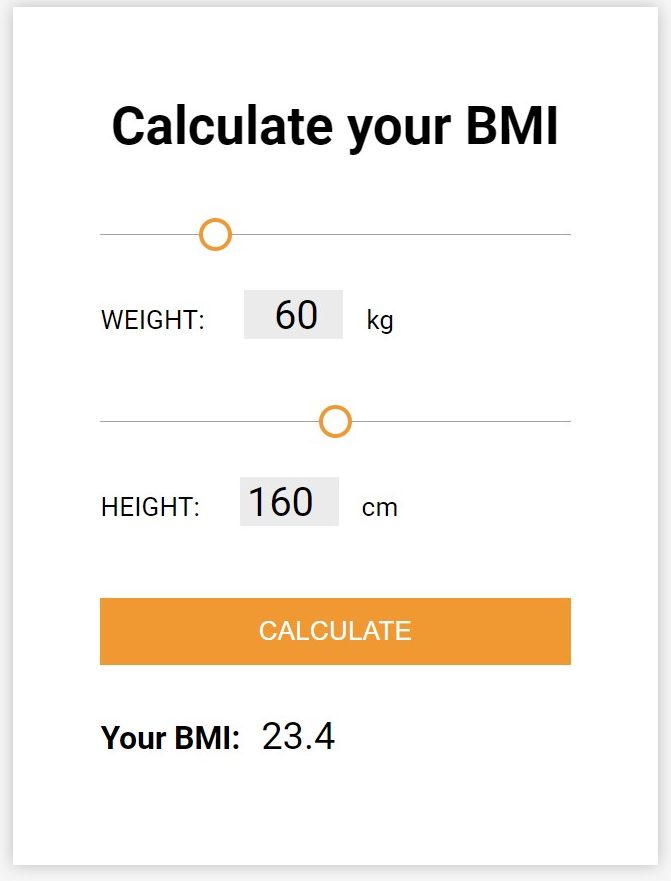
There are two ways to calculate BMI: manually or by entering the values into a BMI calculator and receiving the actual BMI. The formula will be the same in both cases, but manual BMI calculation is a time-consuming process, so we will create our own BMI Calculator to save time.
50+ HTML, CSS &JavaScript Projects With Source Code
There are some criteria that will help you determine which class you belong in, such as underweight, healthy, overweight, and obese. You can better understand it by looking at the chart below.
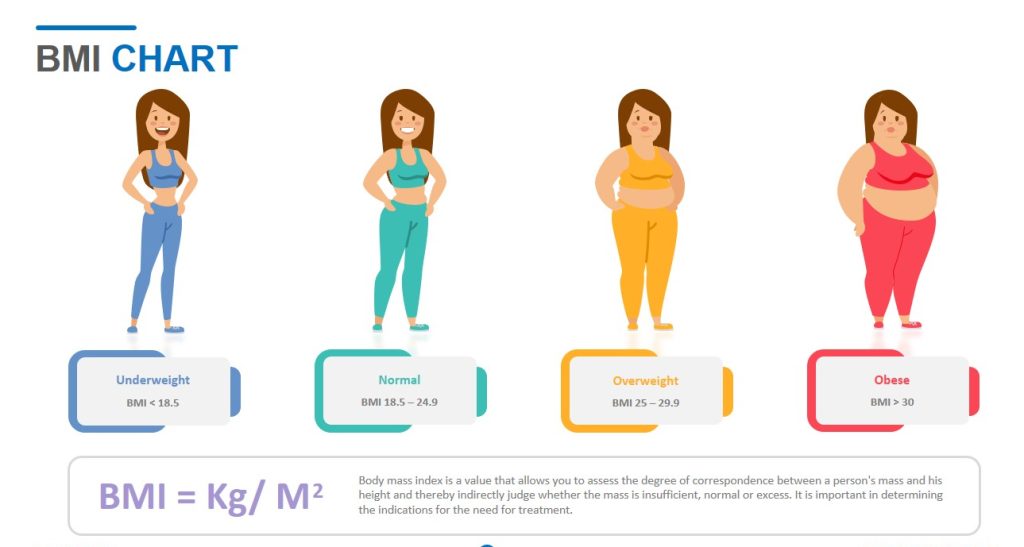
Live Demo
Guys, we’ve also provided you with a live demonstration to help you understand the concept better. We will learn about the entire project from the ground up, which will help beginners understand the concept clearly.
Demo
Hopefully, the demo above has helped you get this project live experience. You can copy the source code needed to create it from above. Also, the download button at the bottom of the article will help.
I hope you got an idea about the project.
Code by | Ian Parkinson |
Project Download | Link Available Below |
Language used | HTML , CSS and JavaScript |
External link / Dependencies | No |
Responsive | Yes |
To complete this project, you must be familiar with HTML and CSS. Create an HTML and CSS file first, then proceed with the steps below.
ADVERTISEMENT
Restaurant Website Using HTML and CSS
ADVERTISEMENT
ADVERTISEMENT
Step1: Making the Calculator’s Basic Structure
ADVERTISEMENT
First and foremost, we used a div tag to create the main container, which will be used to add the basic structure of our calculator.
ADVERTISEMENT
<div class="bmi-calculator"> </div>
Now we’ll use CSS to add the styles needed to our webpage and container to make it look like a calculator.
/* Import Roboto font from Google Fonts */ @import url('https://fonts.googleapis.com/css?family=Roboto:400,500,700&display=swap'); /* Define CSS variables for colors */ :root { --background-color: #f6f6f6; --vigo-color: #f09831; --slider-color: #A3A3A3; --input-number-color: #EBEBEB; } /* Prepare your work table. 😎 */ * { box-sizing: border-box; margin: 0; padding: 0; } /* Push sliders down from <h1> tag */ h1 { text-align: center; margin-left: 0.2em; margin-right: 0.2em; margin-bottom: 0.9em; } /* Define positioning of the elements inside <body> */ html, body { height: 100%; width: 100%; margin: 0; padding: 0; background-color: var(--background-color); color: black; } body { display: flex; justify-content: center; align-items: center; font-family: 'Roboto', sans-serif; } /*
Now we will be adding the calculator look to our webpage . we have added the border , backgorund color to our box.
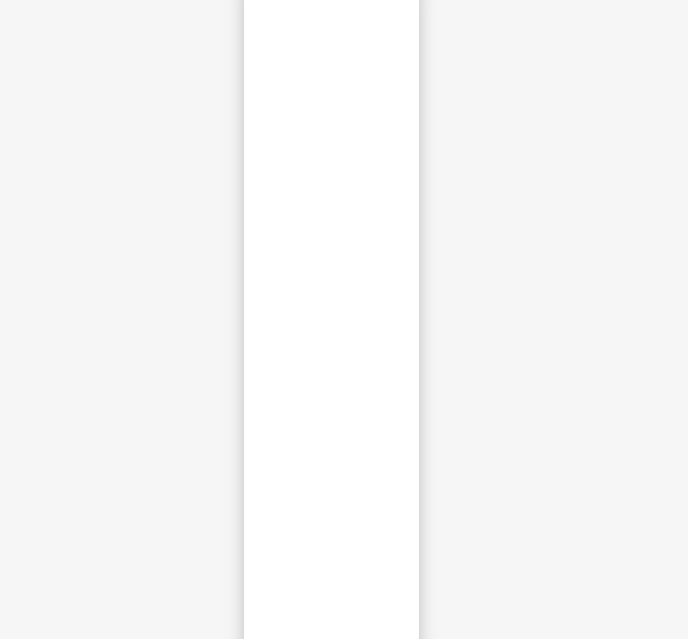
100+ JavaScript Projects With Source Code ( Beginners to Advanced)
Step 2: Adding a heading and slider using HTML
To add a heading to our webpage we have used the h1 tag and added some styles like increasing the font-size and text-alingment property to add the styles to our webpage.
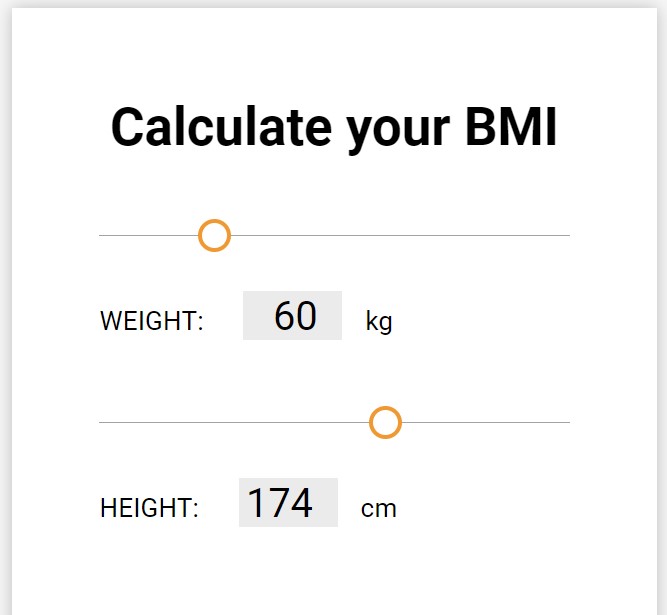
.bmi-calculator-weight, .bmi-calculator-height { /* Positioning */ /*border: dotted 1px red;*/ margin-bottom: 2.2em; width: 100%; /* background: repeating-linear-gradient(90deg, #c8c8c8, #c8c8c8 0.125em /* lines *//*, transparent 0.125em, transparent 1.25em /* space between *//*) 80% no-repeat; /*background-size: 100% /* = 10*1.25em + .125em */ /*20%;*/ } /* Styling for both sliders */ .weight-slider, .height-slider { width: 100%; height: 1px; border-radius: 10px; background: var(--slider-color); /* Light grey background. */ outline: none; /* Remove outline. */ /*opacity: 0.7; *//* Set transparency (for mouse-over effects on hover) */ /*-webkit-transition: .2s;*/ /* 0.2 seconds transition on hover */ /*transition: opacity .2s;*/ }
Step 2: Adding the calculate using HTML
Now using the input with type as “button” we have added the onlick function to our button for the javascript button . Also we have used some style to add the background color and with some width and margin to our input box .
<input class="gumb" type="button" value="Calculate" onclick="calculateBmi()" style="margin-top: 0.5em">
.gumb { text-align: center; font-size: 1em; color: white; width: 100%; margin-bottom: 10px; padding: 11px 32px; border: none; background-color: var(--vigo-color); text-transform: uppercase; cursor: pointer; }
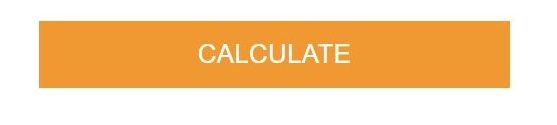
Ecommerce Website Using HTML, CSS, & JavaScript (Source Code)
Step 4: Create a display to view BMI
I’ve now created a display that will show the calculations. There is no set height for this display. Its size will be determined by the amount of content.
<p style="margin-top: 1em;font-size: 1.2em;"> <strong>Your BMI:</strong> <span id="yourbmi" style="font-size: 1.2em;margin-left: 8px;"></span> </p> <p></p> <p> <span id="evaluationMessage"></span> </p>
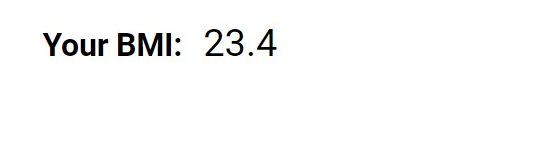
Step5 : Adding the functionality to our calculator
Using HTML and CSS, we created a BMI calculator above. It now needs to be implemented using JavaScript.
Weather App Using Html,Css And Javascript (Weather App Source Code )
We will be defining the variables for range sliders and weight variable and similarly we have added the variables for our height
var weightSlider = document.getElementById("myWeight"); var weightOutput = document.getElementById("inputWeight"); // Height variables var heightSlider = document.getElementById("myHeight"); var heightOutput = document.getElementById("inputHeight");
Now we will be display the slider values using .innerHTML and .value property
weightOutput.innerHTML = weightSlider.value; heightOutput.innerHTML = heightSlider.value;
We also included a real-time slider to update the value. For this, we’ve included an input in which the value of the slider is updated to the inner values of weight and height as needed.
weightSlider.oninput = function () { weightOutput.innerHTML = this.value; } heightSlider.oninput = function () { heightOutput.innerHTML = this.value; }
Now we’ll add the formula for calculating the BMi. First and foremost, we will accept the user’s weight and height, and then we will add that value to the formula. Whatever the value is, we will compare it using the for loop, and then define the person as underweight, healthy, overweight, or obese based on that value.
The value will then be displayed to the user using those values.
function calculateBmi() { var weight = document.bmiForm.realweight.value; var height = (document.bmiForm.realheight.value)/100; var realbmi = (weight)/Math.pow(height, 2); var realbmiOutput = document.getElementById("yourbmi"); var messageOutput = document.getElementById("evaluationMessage"); var roundedBmi = realbmi.toFixed(1); messageOutput.innerHTML = ""; // Clear message before calculating new BMI realbmiOutput.innerHTML = " " + roundedBmi; // Print BMI // Appropriate message for your BMI rating if (roundedBmi > 26) { messageOutput.innerHTML = "YOU'RE A FAT ASS!!!"; } else { } // Console loggings console.log('Teža: ' + weight + " kg"); console.log('Višina: ' + height + " m"); console.log('BMI: ' + realbmi); }
I hope you learned how I made this BMI Calculator using HTML, CSS, and JavaScript from this tutorial. I’ve tried to provide you with complete information, step by step.
Gym Website Using HTML and CSS With Source Code
Now we’ll take a look at our project’s video preview. So, let us look at our project.
Output:
Live Preview Of BMI Calculator using HTML , CSS & Javascript
Now We have Successfully added BMI Calculator using HTML , CSS & Javascript . You can use this project directly by copying into your IDE. WE hope you understood the project , If you any doubt feel free to comment!!
If you find out this Blog helpful, then make sure to search code with random on google for Front End Projects with Source codes and make sure to Follow the Code with Random Instagram page.
Which code editor do you use for this BMI Calculator coding?
I personally recommend using VS Code Studio, it’s straightforward and easy to use.
What is a BMI Calculator?
BMI calculator stands for Body Mass Index. BMI calculator is used to calculate the body mass of an individual . Body mass refers not only to the fat within your body but also within muscles and bones.
What is the formula for Body Mass Index?
Formula: weight (kg) / [height (m)]2
Weight in kilograms divided by square of height in metres is the calculation for BMI. When converting a height measurement from centimetres to metres, reduce the result by 100.