Random Hex Code Generator Using HTML, CSS, and JavaScript
Hi programmers! Today in this article we will learn how you can create a Hex code generator – Fully Responsive. Before starting with the code let us see what Hex code is.
A hex code is a hexadecimal way to represent a color in RGB format by combining three values – the amounts of red, green, and blue in a particular shade of color. These hex codes for colors have been an integral part of HTML for web designing, and are a key way of representing color formats digitally.
Modern technologies use RGB color values and may or may not use hex values to represent them. In general, new users are less familiar with hex color codes than they were in the early days of HTML. However, the newest technologies that work with digital color still acknowledge the representations of colors and hex values.
In this article, we present how to create a Random Hex Code Generator Using HTML, CSS, and JavaScript with complete source code for you so you can copy and paste it into your project.
Now that we know what a Hex color code is, let us start creating a HEX Code Generator which will generate a random color along with its hex code on the button click. We will be using HTML, CSS, and JavaScript for our project.
Step 1: Adding HTML for Code Generator
<html> <head> <title>Hex Code Generator</title> <link rel="stylesheet" href="hexCodeGen.css"> </head> <body> <div class="container"> <h1 class="hexColor">This color HEX code is:</h1> <h3 class="hex"></h3> <button class="nextBtn"> Press Here To change a Color</button> </div> <script src="hexCodeGen.js"></script> </body> </html>
In order to successfully create our search filter we need to connect our HTML, CSS, and Javascript files and this is how we do it:
- Inside the <head> section we will link our CSS file using the link tag.
<head> <title>Hex Code Generator</title> <link rel="stylesheet" href="hexCodeGen.css"> </head>
- And inside the <body> we will add our javascript file using the script tag at the end of our HTML elements.
<script src="hexCodeGen.js"></script>
Now let us get back to the HTML code of our Hex code generator and understand its creation.
- Create a division using a div tag with class=”container”.
- Inside this div tag use, write the heading “This color HEX code is:” using the heading tag. Write class=”hex color” in it.
- Inside another heading tag with class=”hex”, we will insert a button tag and the text we want to display on our button is “Press Here To change a Color”.
Using the above steps we have created the basic structure of our Hex code generator using HTML
Indian Flag Source Code Html And Css | Indian Flag Html CSS
OUTPUT: HTML
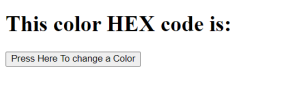
Step 2: Giving basic styling using CSS
Here in this project, we have styled our elements using javascript just to get an idea of how that works. You can style using CSS as well.
CSS Code for Hex Code Generator
*{ margin: 0; padding: 0; } body { text-align: center; }
- Using the universal selector, we will set the margin and padding as 0.
- By targeting the body of our HTML document we will use the text-align property and set it center which will set all the text on our page at the center.
OUTPUT: With HTML and CSS
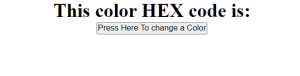
Step 3: Adding Javascript to give logic to our project
Here is the code, you can copy it to directly use it in your IDE for this project. In Javascript, we have also given styling of our project.
const hexNumbers = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 'A', 'B', 'C', 'D', 'E', 'F']; const nextBtn = document.querySelector('.nextBtn'); const bodyBck = document.querySelector('body'); const hex = document.querySelector('.hex'); nextBtn.addEventListener('click', getHex); function getHex() { let hexCol = "#"; for (let i = 0; i < 6; i++) { let random = Math.floor(Math.random() * hexNumbers.length); console.log(random) hexCol += hexNumbers[random]; } bodyBck.style.backgroundColor = hexCol; bodyBck.style.textAlign = 'center'; hex.innerHTML = hexCol; hex.style.fontSize = "3.5rem"; hex.style.fontFamily = "sans-serif"; hex.style.fontWeight = "900"; hex.style.marginTop = "10px"; } // Just for fun lets style everything with Javascript const container = document.querySelector('.container'); container.style.position = 'absolute'; container.style.top = '50%'; container.style.left = '50%'; container.style.transform = "translate(-50%,-50%)"; nextBtn.style.background = "transparent"; nextBtn.style.textTransform = "uppercase"; nextBtn.style.padding = "10px 20px"; nextBtn.style.boxShadow = "0 3px 3px #000"; nextBtn.style.fontWeight = "500"; nextBtn.style.marginTop = "10px";
Gradient background with Waves Using HTML &CSS
Let us understand the Javascript code:-
- Create an array called hex numbers and insert values from 0 to 9 and alphabets in the upper case from A to F. These values will get arranged randomly and then create the 6-digit Hex code for the colors.
- We will now create the following variables using const as we do not want the values of these variables to be changed later on: the next ten will get us a query(class) -next, using dot selector, bodypack will target all the elements inside the body tag and hex will target all the elements with class hex using the dot selector.
- Now we will add an event listener for variable next ten with a click event invoking the get function.
- We will now declare the getHex function and add functionality to it. Inside the function, we will create a variable using const and give the value”#” to it as hex code starts with a #.
- Now, will write code for a loop that generates a random hex code every time we click on the button. As we want 6 values for the array of hex numbers, we will iterate the loop 6 times, i.e from 0 to 6.
- The random variable declared inside the loop will have a value = Math. floor(random number from loop * length of the array.). The Math. floor function will round down and return the largest integer less than or equal to the given number. This will generate a random 6 dig Hex code starting with #.
The code below the logic functionality of the button inside the function is to make changes in the styling on the button click. The following values we have given to the properties to make changes in the styling using Javascript: bodypack. style.backgroundColor = hexCol; bodypack. style.textAlign = ‘center’; hex.innerHTML = hexCol; hex.style.font size = “3.5rem”; hex.style.fontFamily = “sans-serif”; hex.style.font-weight = “900”; hex.style.marginTop = “10px”; .
Coming outside the function, we have given some more styling using Javascript. We have styled all the elements with container class and the next ten.
By writing Javascript and linking it to our HTML document, we have successfully created our Hex code generator. Let us now take a look at the output of our complete project.
OUTPUT: With HTML, CSS, and Javascript Combined.
Before the button click:
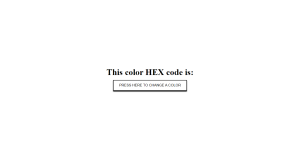
After the Button click: A random color hex code is generated.
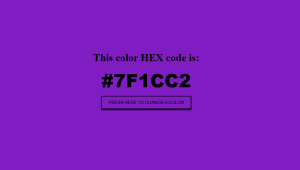
I hope that this article was helpful and you understood the whole project. Now let’s take a look at the Live preview of Hex Code Generator.
Create Instagram Logo Using HTML CSS (Source Code)
Now we have successfully created our Hex Code Generator using HTML, CSS & JavaScript. You can use this project directly by copying it into your IDE. We hope that you understood the project, If you have any doubts then feel free to comment!!
ADVERTISEMENT
In this article, we present a Random Hex Code Generator Using HTML, CSS, and JavaScript with complete source code for you so you can copy and paste it into your project.
ADVERTISEMENT
I hope you got some idea about random hex Code Generator with simple use language Html and CSS.
ADVERTISEMENT
Thank You And Happy Learning!!!
ADVERTISEMENT