Responsive Product Card Using HTML & CSS
Hello, We’ll demonstrate today how to create a fully Responsive Product Card page using simply HTML and CSS. A product’s image or photo that is connected in some way to the associated products is displayed on the product card. It provides information on the product’s specifications.
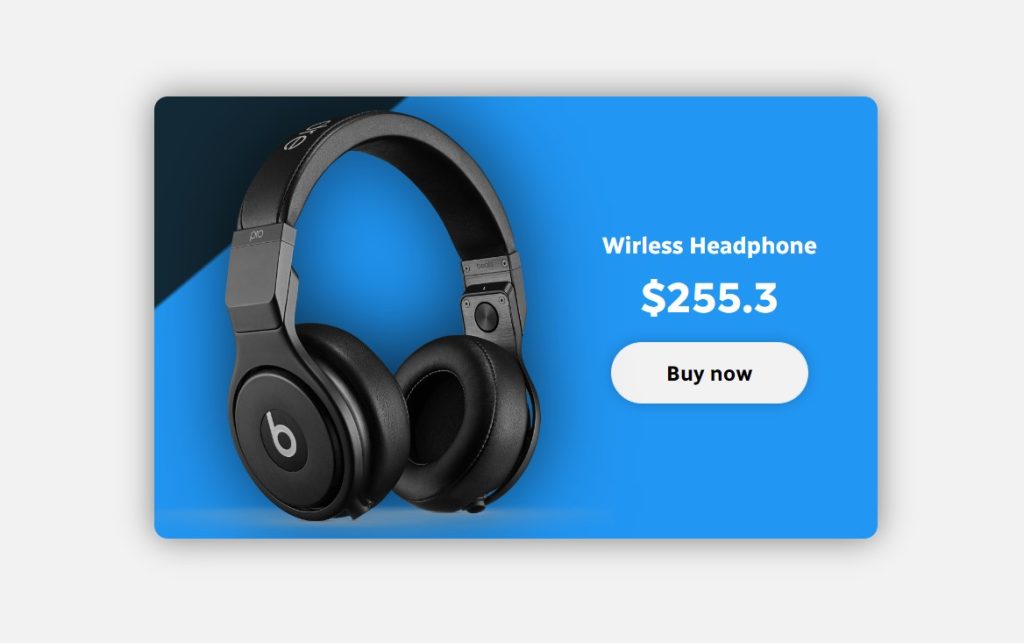
The product card on this blog is divided into two sections: the left section features a product image, and the right section includes the product name, price, and buy now button.
You can copy the source code of this application if you wish it; it is provided below with the product card design. This product card design code, along with your creativity, can be used to elevate this product card.
Step1: Adding HTML Markup
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta http-equiv="X-UA-Compatible" content="IE=edge" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <link rel="stylesheet" href="style.css" /> <title>Product Card</title> </head> <body> <section class="section-card"> <div class="card"> <div class="img-container"> <img src="https://www.downloadclipart.net/large/sony-headphone-png-photos.png" alt=""> </div> <div class="infos"> <h3 class="name"> Wirless Headphone </h3> <h2 class="price"> $255.3 </h2> <button class="btn btn-buy">Buy now</button> </div> </div> </section> </body> </html>
We will create two separate HTML and CSS files in our code editor to ensure optimal code organisation. HTML and CSS will be used to construct our login form. Add the CSS link to our HTML now.
<link rel="stylesheet" href="style.css" />
Adding the Structure for our Product Card:
- We’ll make a section with the class “section-card” using the <section> tag, then we’ll make the container for the card using the div tag.
- We’ll make a container for the image inside our card container and, using the <img> tag, we’ll add an image of the item inside our card.
- The <h3> tag will now be used to include the product details. The product’s name will be added, and the price will be added with the <h2> tag. We’ll also include a buy now button inside our product card utilising the button tag.
Movie App using HTML, CSS, and Javascript
Let’s have a look at the structure.
Output:
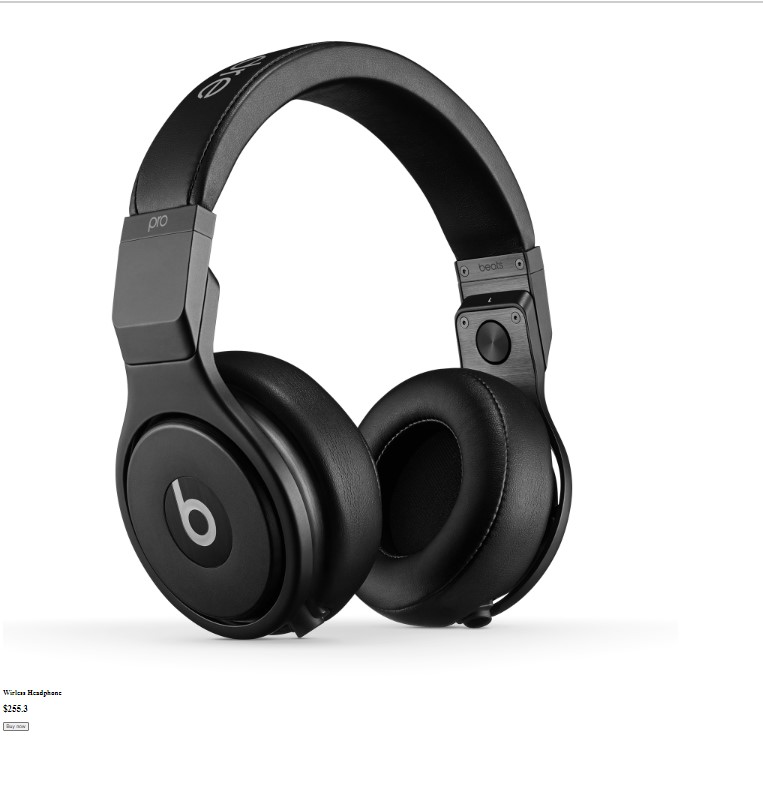
Step2: Adding CSS Code
In your stylesheet, copy and paste the CSS code provided below.
@import url("https://fonts.googleapis.com/css2?family=Radio+Canada:wght@700&display=swap"); * { margin: 0; padding: 0; box-sizing: border-box; } body { font-family: "Radio Canada", sans-serif; background-color: #f2f2f2; } img { width: 100%; display: block; } .section-card { padding: 0 30px; height: 100vh; display: flex; align-items: center; } .section-card .card { margin: 20px auto; max-width: 300px; border-radius: 10px; overflow: hidden; background-image: linear-gradient( 140deg, #132936, #132936 20%, #2196f3 20.5%, #2196f3 ); box-shadow: 0 0 25px rgba(0, 0, 0, 0.5); } .section-card .card .img-container { padding: 10px 0 0 0; } .section-card .card .img-container img { filter: drop-shadow(0px -5px 25px rgba(0, 0, 0, 0.7)); transform: scale(1.15); } .section-card .card .infos { padding: 30px 15px; text-align: center; } .section-card .card .infos .name, .section-card .card .infos .price { color: #fff; } .section-card .card .infos .name { font-size: 1.1rem; } .section-card .card .infos .price { margin: 10px 0 15px 0; font-size: 2rem; } .section-card .card .infos .btn { font-family: "Radio Canada", sans-serif; padding: 0.8rem 1.6rem; border-radius: 50px; border: 1px solid #f2f2f2; font-size: 1rem; font-weight: 300; min-width: 150px; cursor: pointer; box-shadow: 0 0px 10px rgba(0, 0, 0, 0.2); background-color: #f2f2f2; transition: all 0.2s ease-in; } .section-card .card .infos .btn:hover { background-color: transparent; color: #fff; box-shadow: none; } @media screen and (min-width: 700px) { .section-card .card { display: flex; align-items: center; min-width: 550px; padding: 15px 20px; } .section-card .card .img-container { padding-top: 0; width: 60%; } }
Basic Styling:
The Google fonts will first be imported using the Google font import link, after which the padding and margin will be set to “zero” using the universal selector, and the box-sizing property will be used to change the box’s size to “border-box.”
Now, inside the body, we will use the body tag selector to set the font family to “Radio Canada” and the background colour property to gray. The image’s width is set to 100%, and its display is set to “block.”
@import url("https://fonts.googleapis.com/css2?family=Radio+Canada:wght@700&display=swap"); * { margin: 0; padding: 0; box-sizing: border-box; } body { font-family: "Radio Canada", sans-serif; background-color: #f2f2f2; } img { width: 100%; display: block; }
Styling Card:
We will give our card a padding of 30px using the class selector (.section-card), a height of 100vh using the height property, and the display setting of “flex.” We will align the objects using the align-item attribute.
50+ HTML, CSS & JavaScript Projects With Source Code
The border radius will now be set to 10px using the child selector (.card), the overflow property will be set to “hidden,” and the background image will be used to establish the background as a linear gradient of “black and blue.”
.section-card { padding: 0 30px; height: 100vh; display: flex; align-items: center; } .section-card .card { margin: 20px auto; max-width: 300px; border-radius: 10px; overflow: hidden; background-image: linear-gradient( 140deg, #132936, #132936 20%, #2196f3 20.5%, #2196f3 ); box-shadow: 0 0 25px rgba(0, 0, 0, 0.5); }
Styling Information:
For that, we will add padding of 30 px from the top and bottom and 15 px from the left and right using the class selector (.infos), and the font colour will change to “grey.” We will set the font size to “2 rem” using the font-size attribute.
Our button will have a 1 pixel solid grey border when styling is applied. Using the background colour property, we will give our button a grey background and an order-radius of 50px to create a curvature to the edges.
We will use the hover property to give our button a hover property so that when the user hovers over it, the background colour turns transparent and the button’s font colour is set to white.
.section-card .card .infos { padding: 30px 15px; text-align: center; } .section-card .card .infos .name, .section-card .card .infos .price { color: #fff; } .section-card .card .infos .name { font-size: 1.1rem; } .section-card .card .infos .price { margin: 10px 0 15px 0; font-size: 2rem; } .section-card .card .infos .btn { font-family: "Radio Canada", sans-serif; padding: 0.8rem 1.6rem; border-radius: 50px; border: 1px solid #f2f2f2; font-size: 1rem; font-weight: 300; min-width: 150px; cursor: pointer; box-shadow: 0 0px 10px rgba(0, 0, 0, 0.2); background-color: #f2f2f2; transition: all 0.2s ease-in; } .section-card .card .infos .btn:hover { background-color: transparent; color: #fff; box-shadow: none; }
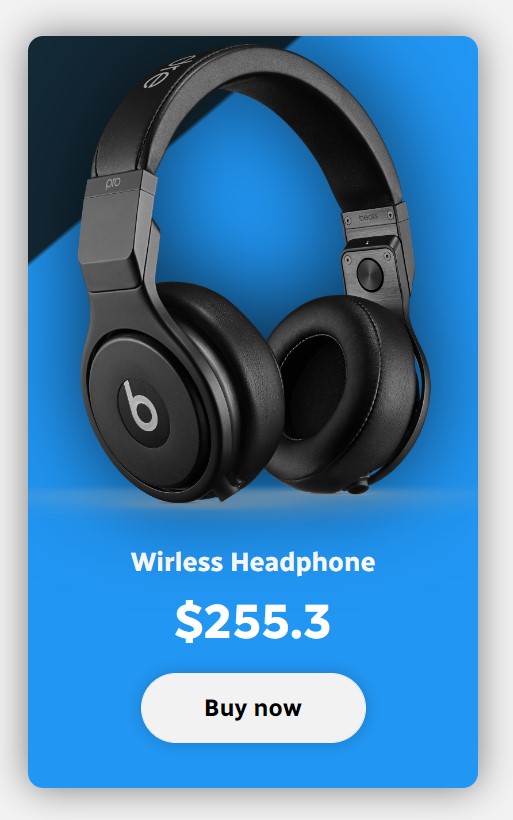
Responsiveness:
We will now use the media query to add responsiveness to our product. If the screen size is equal to or more than the stated width, we shall set a minimum width. When the screen size changes, our card display will switch to flex and our product card will be given a minimum width of 550 pixels. Additionally, we will add padding that is 20 pixels on the left and right and 15 pixels from the top.
ADVERTISEMENT
@media screen and (min-width: 700px) { .section-card .card { display: flex; align-items: center; min-width: 550px; padding: 15px 20px; } .section-card .card .img-container { padding-top: 0; width: 60%; } }
Now we’ve completed our product card using html & css. I hope you understood the whole project. Let’s take a look at our Live Preview.
ADVERTISEMENT
Portfolio Website using HTML and CSS (Source Code)
ADVERTISEMENT
Output:
ADVERTISEMENT
Live Preview Of our product card using Html & Css
Now We have Successfully created our product card using html & css. You can use this project directly by copying into your IDE. WE hope you understood the project , If you any doubt feel free to comment!!
ADVERTISEMENT
If you find out this Blog helpful, then make sure to search code with random on google for Front End Projects with Source codes and make sure to Follow the Code with Random Instagram page.