Let us make a QR Code generator using HTML, CSS, and JavaScript.
Hello Programmer! Today we are going to create a QR Code Generator Using HTML, CSS, and JavaScript.
What is a QR code generator?
A QR code generator is an application that stores any required textual data into a QR code which can be later scanned with a QR code scanner to reveal the stored information.
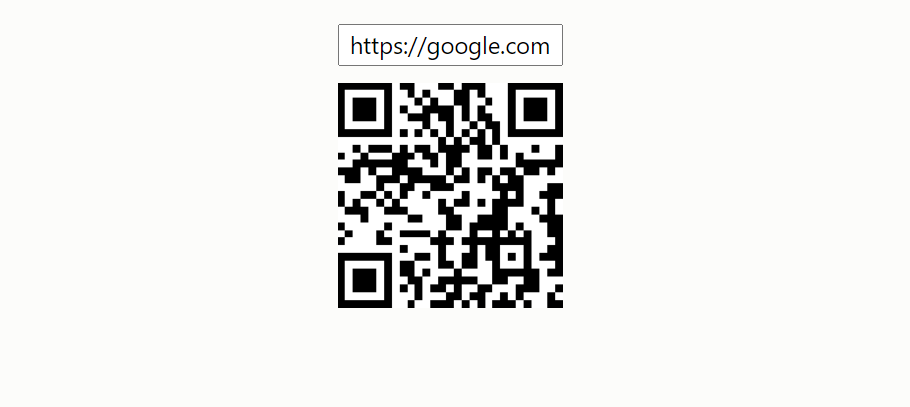
Need of HTML QR Code
This QR Code can be used anywhere, for example, on a poster or website to allow users to get additional information. This application will allow the user to type in the data required and save a PNG or SVG image of the QR code.
While you can generate QR codes for URLs in browsers such as Chrome, it’s always interesting to learn how you can make your own version of a simple QR code generator. So, here we go.
Code by | Eitan |
Project Download | Link Available Below |
Language used | HTML, CSS and JavaScript |
External link / Dependencies | Yes |
Responsive | Yes |
QR Code is becoming very popular with more and more applications using it for information scanning a QR code is a machine-readable barcode that can be used to hide information from humans and can be accessed via scanning from a mobile application.
Build a QR Code Generator in 3 Easy Steps
- Create the Layout of the QR Code generator and Step Elements Using HTML.
- Make Functional Using JavaScript.
- Design the QR Code generator and the Step Elements Using CSS.
You Might Like This:
- Navbar Hover Effect Using Html And Css
- Horizontal Timeline Using Html Css Javascript
- Indian Flag Source Code Html And CSS
- Flip Card Responsive Css
- Restaurant Website Using HTML and CSS
- 3D Card Rotate On Mouseover
- 50+ Frontend Project CodeĀ
HTML Code:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta http-equiv="X-UA-Compatible" content="IE=edge" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Qr Code Generator</title> <link rel="stylesheet" href="https://fonts.googleapis.com/css2?family=Ubuntu&display=swap" /> <link rel="stylesheet" href="./style.css" /> <script src="https://cdnjs.cloudflare.com/ajax/libs/qrcodejs/1.0.0/qrcode.min.js"></script> </head> <body> <div class="header"> <div class="box"> <h1>QR Code Generator</h1> <hr /> <div class="sqrcode"></div> <div class="qrcode"></div> <input type="text" placeholder="Paste an URL or enter text, then press enter" onchange="generateQr()" /> </div> </div> <script src="./app.js"></script> </body> </html>
Here’s a quick look at the HTML code and it’s pretty straightforward. The last element is for the QR code to be displayed as soon as we fetch it from a library through JavaScript (more on that later).
The code is a webpage with the following code:
ADVERTISEMENT
ADVERTISEMENT
The head section contains meta tags that specify how to display the page in different browsers and what character set is used on the page (UTF 8). The link tag specifies where to find style sheets for this website.
ADVERTISEMENT
ADVERTISEMENT
The code is a head tag that includes the meta tags, which are used to provide information about the site. The next line of code is an HTML link tag that points to a CSS file. This CSS file will be used for styling purposes. Next, there is a script tag that loads qrcodejs library from cdnjs and provides it with the name of the JavaScript file in which this snippet resides.
ADVERTISEMENT
The code is a div with two other divs inside of it. The first one is the header, which has a box that says “QR Code Generator” and then an hr tag. Inside of this is another div called sqrcode, which contains a QR code image. Then there’s qrcode, which also contains a QR code image but in different colors.
There’s also an input field where you can paste text or enter your URL to generate the QR code for you. The script tags are used to load app.js into the page so that it can be executed when someone clicks on the button labeled “generateQr()”. The code will generate a QR code with the text “Paste a URL or enter text, then press enter” and a button that when clicked on will generate the QR code.
The generated QR code is placed in the div element with class=”qrcode”.
HTML Output
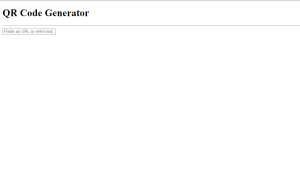
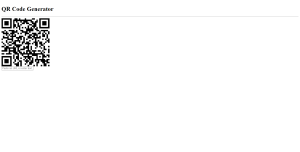
JavaScript code:
var qrcode = new QRCode(document.querySelector(".qrcode")); qrcode.makeCode("Why did you scanned me?"); function generateQr() { qrcode.makeCode(document.querySelector("input").value); }
The code starts by creating a new QRCode object. The makeCode() method takes in the text to be encoded and returns an array of characters that are then displayed on the screen. The generateQr() function is used to create QR codes for input values from the user. The code creates a QR code with the text “Why did you scanned me?” on it. The function generateQr() is called when the user scans the QR code and this function makes a new QR code with the inputted text.
CSS Code
* { margin: 0; padding: 0; box-sizing: border-box; } html { background: linear-gradient(125deg, orange, purple); min-height: 100vh; font-family: "Ubuntu", sans-serif; } .header { display: flex; align-items: center; justify-content: center; min-height: 100vh; } .header .box { background: #f4f4f4; padding: 20px; border-radius: 20px; color: #1e7fb0; box-shadow: 10px 10px rgba(0, 0, 0, 0.2); text-align: center; } .header .box hr { border-top: 2px solid #a2a2a2; margin: 3px; } .header .box input { width: 100%; margin-bottom: 3px; height: 50px; padding: 3px; resize: vertical; } .header .box .qrcode { padding: 3px; } .header .box .sqrcode { padding: 3px; margin-left: 15px; } .header .box button { width: 100%; height: 35px; background: #1e7fb0; font-weight: 700; font-family: "Ubuntu", sans-serif; border-radius: 5px; border: none; cursor: pointer; }
The code starts with a tag and then has some CSS code. The first thing that is done in the CSS is to set the background of the page to be linear-gradient(125deg, orange, purple). This means that there will be an orange gradient on top of a purple background. Next, it sets min-height: 100vh; which means that the height of all elements should be at least 100 pixels.
Then it sets the font family : “Ubuntu”, sans-serif; which means Ubuntu Sans Serif as the default font for text on this website. Next comes some HTML code where we have two divs with different classes applied to them. One class called .header has its display property set to flex and align-items property set to center so that they are centered horizontally across their parent container. The other div called .box has its display property set to box and justify-content property set to center so that it is centered vertically within its parent container.
The code will produce the following outcome: The header is centered and has a width of 100vh. The header is surrounded by a border with a 20px radius. The background color of the header is #f4f4f4, which has been set to purple. A box shadow has been added to the top left corner of the header with 10px height and 10px width. The text in the center of the box is bolded and centered horizontally on top of it.
The code is a header for the website. It has a box with an input and button. The input is used to enter text, while the button is used to submit that text. The code is a CSS style sheet that sets the header of the website to have a box-like shape. The header also has a button on it, which is styled with CSS and HTML. The code above styles the header’s button so that it has rounded corners, a white background, and some text on top of it.
Final Output
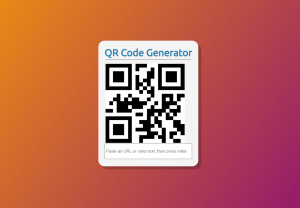
Live Preview Of QR Code GeneratorĀ
You have completed learning QR code generator using html css js
If you enjoyed reading this post and have found it useful, then please share it with your friends, and follow us to get updates on my upcoming posts.Ā You can connect with me onĀ Instagram.
If you have any confusion Comment below or you can contact us by filling out our Contact Us form from the home section. š¤š
Code By ā Eitan
written by āĀ Ninja_webTech
Some FAQs :
Which code editor do you use for this QR Code generator coding?
I personally recommend using VS Code Studio, it’s straightforward and easy to use.
is this project responsive or not?
YES! This is a responsive project
Do you use any external links to create this project?
Yes!