How to Create a Dynamic Card Using JavaScript?
Hello Coder! Welcome to today’s tutorial on codewithrandom. We’ll learn how to make dynamic cards using JavaScript. We create a color-changing dynamic card where we can select a color, and that color is applied to our dynamic card.
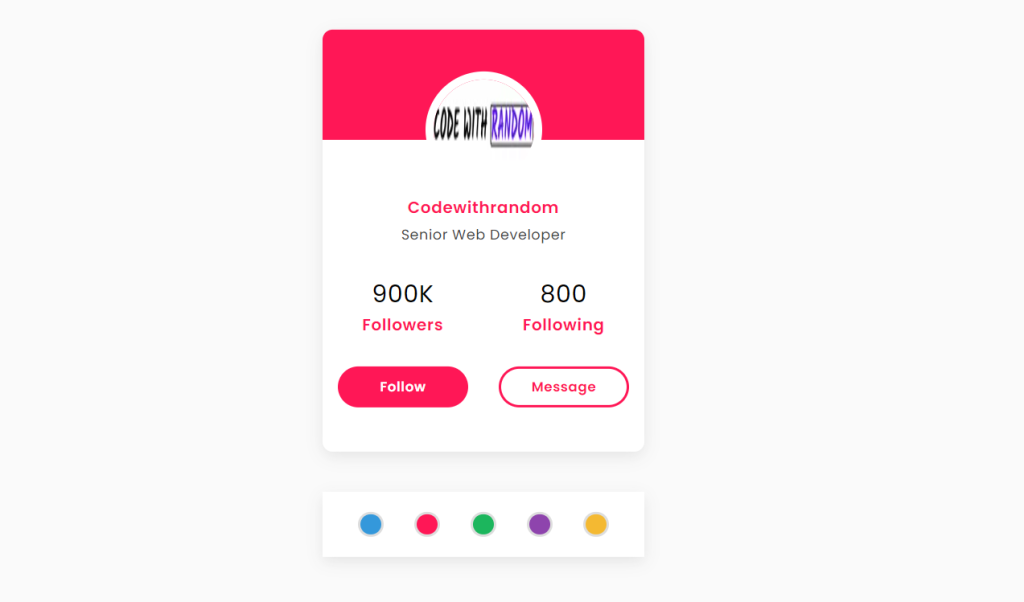
Before we dive into the project, let’s understand some of the major concepts of dynamic cards.
What is a Dynamic Card Javascript?
A dynamic card CSS is a unique type of card that contains user data and information and displays it in the contact form format. Using the dynamic javascript function, we will change the color of the dynamic card using a button click.
What is the benefit of using Dynamic Card Javascript?
The benefit of using Dynamic Card is:
- Enhance User Interaction.
- Improves content readability.
- Grabs User attention.
50+ HTML, CSS & JavaScript Projects With Source Code
The HTML (Hypertext Markup Language) will help us create the structure for the list with some necessary attributes and elements.
Then we will use CSS (Cascading Stylesheet), which will help us style or design the project with suitable padding and alignment.
At last, we will use JS (JavaScript), which will add logic to make the project responsive from the user’s end.
I hope you have got an idea about the project.
Dynamic Card HTML Code:-
<html lang="en"> <head> <title>Card With Dynamic Themes</title> <!--Google Font--> <link rel="preconnect" href="https://fonts.gstatic.com"> <link href="https://fonts.googleapis.com/css2?family=Poppins:wght@400;600&display=swap" rel="stylesheet"> <!--Stylesheet--> <link rel="stylesheet" href="style.css"> </head> <body> <div class="container"> <div class="card"> <img src="https://i.postimg.cc/ZqRhqsfr/1.webp"> <h4>Codewithrandom</h4> <h5>Senior Web Developer</h5> <div class="details"> <div class="column"> <h2>900K</h2> <span>Followers</span> </div> <div class="column"> <h2>800</h2> <span>Following</span> </div> </div> <div class="buttons"> <button>Follow</button> <button>Message</button> </div> </div> <div class="themes"> <button class="btn btn1"></button> <button class="btn btn2"></button> <button class="btn btn3"></button> <button class="btn btn4"></button> <button class="btn btn5"></button> </div> </div> <!--Script--> <script src="script.js"></script> </body> </html>
First, we’ll start with creating the structure of the project. As you can see in the above code, we have used all the necessary elements and attributes. Let us know how to code the CSS part to add styling and align the tags.
Output:
5+ HTML CSS Projects With Source Code
CSS Code for Dynamic Card:-
*, *:before, *:after{ padding: 0; margin: 0; box-sizing: border-box; } :root{ --theme-color: #ff1756; } body{ background-color: #fafafa; } .container{ position: absolute; transform: translate(-50%,-50%); top: 50%; left: 50%; } .card{ height: 420px; width: 320px; background: linear-gradient( to bottom, var(--theme-color) 110px, #ffffff 110px ); border-radius: 10px; box-shadow: 0 5px 15px rgba(50,50,50,0.1); padding: 50px 0; } .card *{ font-family: 'Poppins',sans-serif; text-align: center; letter-spacing: 0.5px; font-weight: 600; } img{ display: block; width: 100px; height: 100px; position: relative; border-radius: 50%; margin: 0 auto; box-shadow: 0 0 0 8px #ffffff; } .card h4{ color: var(--theme-color); font-size: 16px; margin: 15px 0 5px 0; } .card h5{ color: #454545; font-weight: 400; font-size: 14px; } .details{ width: 100%; margin-top: 30px; display: flex; justify-content: space-around; } .details h2{ font-weight: 400; } .details span{ color: var(--theme-color); } .buttons{ width: 100%; display: flex; justify-content: space-around; margin-top: 30px; } .buttons button{ width: 130px; padding: 8px 0; border-radius: 25px; border: 3px solid var(--theme-color); } .buttons button:first-child{ background-color: var(--theme-color); color: #ffffff; } .buttons button:last-child{ background-color: transparent; color: var(--theme-color); } .themes{ background-color: #ffffff; box-shadow: 0 5px 15px rgba(50,50,50,0.1); padding: 20px; margin-top: 40px; display: flex; justify-content: space-around; } .themes button{ height: 25px; width: 25px; border: 3px solid #dddddd; outline: none; border-radius: 50%; cursor: pointer; } .btn1{ background-color: #3498db; } .btn2{ background-color: #ff1756; } .btn3{ background-color: #1cb65d; } .btn4{ background-color: #8e44ad; } .btn5{ background-color: #f4b932; }
Second, comes the CSS code which we have styled for the structure we have padded as well as aligned the project so that it is properly situated and doesn’t get messy with suitable CSS elements. Now let’s code the JavaScript part to make it functional.
Output:
Portfolio Website using HTML and CSS (Source Code)
Dynamic Card JavaScript Code:-
const theme = document.querySelector(':root'); const btns = document.querySelectorAll('.btn'); btns.forEach(function(btn){ btn.addEventListener("click", function(e){ const color = e.currentTarget.classList; if(color.contains("btn1")){ theme.style.setProperty("--theme-color", "#3498db"); } else if(color.contains("btn2")){ theme.style.setProperty("--theme-color", "#ff1756"); } else if(color.contains("btn3")){ theme.style.setProperty("--theme-color", "#1cb65d"); } else if(color.contains("btn4")){ theme.style.setProperty("--theme-color", "#8e44ad"); } else{ theme.style.setProperty("--theme-color", "#f4b932"); } }); });
The last stage of the project was JavaScript, in which we added the logic and coded as per the requirements with some conditions. Also, we have defined a function to make the colors responsive to the change in color when the user clicks on them. Let us see the final output of the project. Creating a Dynamic Card using HTML, CSS, and JavaScript (Source Code) | Profile Card UI With Dynamic Theme Colors
100+ JavaScript Projects With Source Code ( Beginners to Advanced)
Final Output Of Dynamic Card Using JavaScript:-
We have successfully created our Creating a Dynamic Card using JavaScript. You can use this project for your personal needs and the respective lines of code are given with the code pen link mentioned above.
ADVERTISEMENT
Restaurant Website Using HTML and CSS
ADVERTISEMENT
If you find out this Blog helpful, then make sure to search Codewithrandom on Google for Front End Projects with Source codes and make sure to Follow the Code with Random Instagram page.
ADVERTISEMENT
Written By – Harsh Sawant
ADVERTISEMENT
Code By – @harshh9
ADVERTISEMENT
What is a Dynamic Card Using Javascript?
A dynamic card is a unique type of card that contains user data and information and displays it in the contact form format. Using the dynamic javascript function, we will change the color of the dynamic card using a button click.
What is the benefit of using Dynamic Card?
The benefit of using Dynamic Card is:
Enhance User Interaction.
Improves content readability.
Grabs User attention.