React is the most widely used framework of javascript used to make dynamic and interactive webpages. React components allow for code reusability and modularity which helps in using the same code just by customizing it for the different projects.
Even though React has a wide range of built-in hooks, you could need your own logic that can be used by several components. Custom React hooks can be useful in this situation.
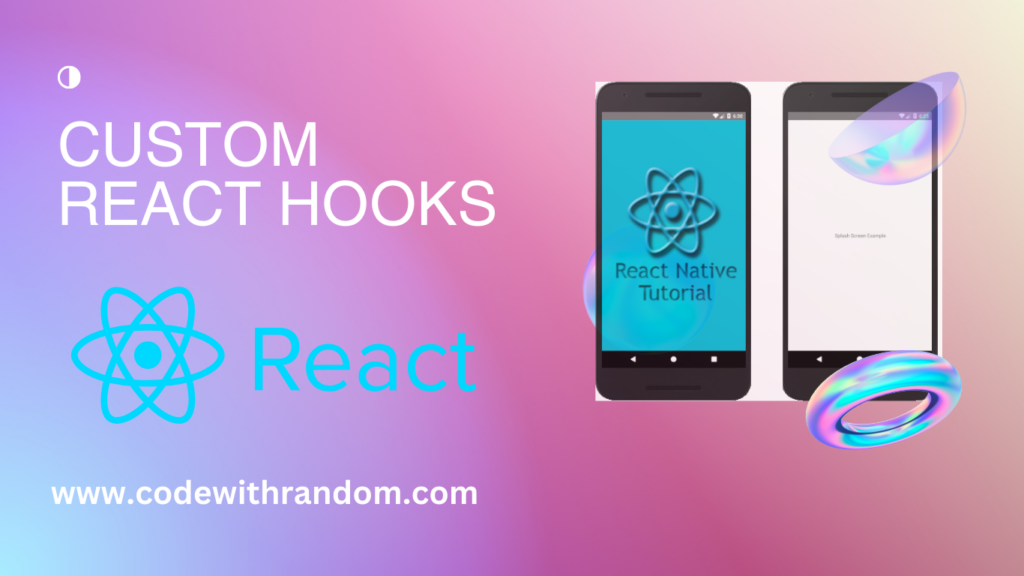
We will learn to create custom hooks in React, their advantages, and how to make them using real-world applications.
Understanding React Hooks
Let’s quickly go over what React hooks are and how they operate before getting into custom hooks. As a mechanism to provide state and side effects to functional components, React introduced hooks in version 16.8. Through the use of hooks, developers may more easily control component states, access the component lifecycle, and arrange complex functionality.
React comes with a number of built-in hooks, including useState, useEffect, useContext, and useReducer. These hooks give developers the ability to handle complicated state management, conduct side effects (such as data fetching), access context, and manage local component states.
Now we will look at the concept of why we actually need custom hooks
Need For Custom Hooks
There may be times when you need to contain a specific piece of logic that is applied to several different components. Custom hooks can be made to encourage reuse and maintainability rather than repeating this functionality in every component or making higher-order components (HOCs).
Custom hooks make it simple to exchange functionality between various areas of your application or even between separate projects by abstracting away the implementation specifics of your logic. As a result, the development process may become more effective and the code may become cleaner and easier to maintain.
Creating a Custom Hook
A custom hook is easy to make and follows a straightforward convention: to identify them as hooks, they should begin with the word “use”. Let’s walk through the steps of developing a custom hook using an actual example.
Step 1: Identify Reusable Logic
The reusable logic you want to incorporate must be identified before you can develop a custom hook. Consider an example in which you have a number of components that must control the loading and error updates while obtaining data from an API.
Step 2: Create the Custom Hook
Here is a fundamental format for developing a unique hook:
import { useState, useEffect } from 'react';
function useDataFetching(url) {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
async function fetchData() {
try {
const response = await fetch(url);
const result = await response.json();
setData(result);
} catch (err) {
setError(err);
} finally {
setLoading(false);
}
}
fetchData();
}, [url]);
return { data, loading, error };
}
export default useDataFetching;
we’ve created a custom hook called useDataFetching. It takes a URL as an argument and returns an object containing data, loading, and error states.
Data fetching in functional components is made simpler via a unique React hook called useDataFetching. Using React’s useState hook, it sets up the data, loading, and error states.
Then, inside a useEffect, it retrieves data asynchronously from a given URL, changing the states as necessary. This hook’s purpose is to provide a straightforward interface for handling data loading and error statuses while also encapsulating the functionality necessary to expedite data fetching operations within functional components.
Step 3: Use the Custom Hook in Components
We can utilize our custom hook in the functional components that we have generated. Here’s an example of useDataFetching in action in a component:
import React from 'react';
import useDataFetching from './useDataFetching';
function DataFetcher({ url }) {
const { data, loading, error } = useDataFetching(url);
if (loading) {
return <div>Loading...</div>;
}
if (error) {
return <div>Error: {error.message}</div>;
}
return (
<div>
<h1>Data</h1>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
);
}
export default DataFetcher;
In this example, data is fetched from a URL by the DataFetcher component using the useDataFetching hook. It’s simple to use this logic in other areas of your application because it takes care of the loading and error statuses.
Step 4: Reuse Across Components
Custom hooks have the advantage of being reusable across several components. Let’s say you have a different component that likewise requires data to be fetched from a different API endpoint. UseDataFetching can be easily imported and used in that component as well.
import React from 'react';
import useDataFetching from './useDataFetching';
function AnotherComponent() {
const { data, loading, error } = useDataFetching('/api/another-endpoint');
// Render and handle data, loading, and error states as needed
}
Benefits of Custom Hooks
Custom hooks come with a number of advantages that make them an effective tool in your React development toolbox:
- Reusability: By including complex functionality and reusing it in different components, you can cut down on code duplication.
- Reusability: By including complex functionality and reusing it in different components, you can cut down on code duplication.
- Testing: Independent tests of custom hooks can confirm the dependability of your application logic.
- Shareable: By sharing personal hooks with the open-source community or other projects, you help strengthen the React ecosystem.
Points to be Remember for Custom Hooks:
- Follow the Hook Naming norm: Use the word “use” at the beginning of your custom hook names to follow the standard and make it obvious that they are hooks.
- Keep Hooks Simple: Try to design unique hooks with just one task. They become more adaptable and simple to comprehend as a result.
- Test Thoroughly: Do proper tests for your unique hooks to make sure they function in the way you want and continue to be reliable.
- Consider Dependencies: Keep in mind the dependencies that your custom hook needs. Document these specifications if your hook depends on a certain release of React or other libraries.
Live Codepen Examples:
Conclusion
To capture and reuse logic in your functional components, utilize custom React hooks. our code can become more modular, maintainable, and shareable by using custom hooks. As a result, the next time our components include complex logic or you find yourself duplicating code, think about developing a custom hook to streamline and organize your codebase.
If you find out this Blog helpful, then make sure to search Codewithrandom on Google for Front End Projects with Source codes and make sure to Follow the Code with Random Instagram page.
Follow: CodewithRandom
Author: Arun