A data type is an attribute of a variable that interacts with the compiler or interpreter of the programmer’s planned usage of the variable. You will learn a little bit about the various data types in this post. Data types can be categorized into two groups based on the characteristics they each possess:
1. Primitive Data type
2. Non-primitive data type
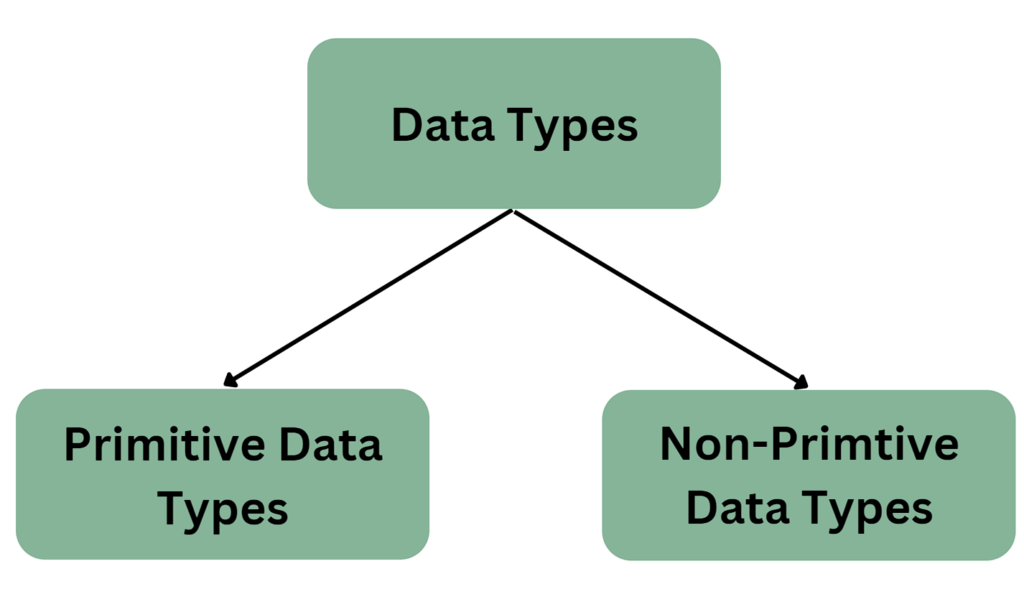
Primitive Data Types: Primitive data types are data types that are already defined inside the programming language. The size and type of the variable are defined inside these datatypes.
Non-Primitive Data Types: The programmers themselves define these data types rather than the programming language. Due to the fact that they make a memory region where the data is stored reference, they are also known as “reference variables” or “object references.”
Primitive Data Types
Primitive data types, often known as basic data types, are the essential building elements of a computer language. In Java, data types are grouped into four categories: int, float, character, and boolean. However, in general, there are eight data types.
- Integer (Int): This data type represents whole numbers that can be both positive and negative. Examples are -1, 0, and 42.
- Floating-Point (float and double): Real numbers with decimal points are represented by these data types. The precision of floats is lower than that of doubles. eg: 3.14, 2.71828.
- Boolean(Bool): Booleans have only two possible values, true or false, and are often used in conditional statements and logic operations.
- Character (char): A single character, such as ‘a’, ‘1’, or ‘$’, is stored in the Char data type.
- Byte: A byte is the smallest unit of memory and can hold values ranging from 0 to 255.
- Short and Long: These data types are used for numbers with variable ranges. Short has a lesser range than int, while long can store larger integers.
- Text and binary data primitive data types: Depending on the programming language, there can be data types such as byte, char, and short can be used to store binary data such as images or files.
Example:
# Integer (int) Example
num1 = 42
print("Integer Value:", num1)
print("Data Type:", type(num1))
# Floating-Point (float) Example
pi = 3.14159
print("\nFloating-Point Value:", pi)
print("Data Type:", type(pi))
# Boolean (bool) Example
is_python_fun = True
print("\nBoolean Value:", is_python_fun)
print("Data Type:", type(is_python_fun))
# Character (str) Example
first_letter = 'A'
print("\nCharacter (String) Value:", first_letter)
print("Data Type:", type(first_letter))
Non-Primitive Data Types
Non-primary data types refer to objects and are hence referred to as reference types. Strings, Arrays, Classes, Interfaces, and so on are non-primitive types.
- Array: Arrays are collections of pieces of the same data type that are arranged in a single contiguous block of memory. They enable you to save multiple values of the same kind under the same variable name.
- Strings: Strings are character sequences that are frequently implemented as classes in several computer languages. They give a number of string manipulation methods.
- Objects and Classes: In object-oriented programming languages such as Java, C++, and Python, objects and classes are used to create complicated data structures that include both data and methods for operating on that data.
- Lists, Sets, and Maps: These are dynamic data structures that allow you to store collections of elements. Lists retain an ordered sequence of elements, sets keep unique elements, and maps associate keys with values.
- Pointers and references: In programming languages such as C and C++, pointers and references are used to store memory addresses, allowing direct access to memory regions. While they are strong, they may contain memory-related flaws.
Example:
# List (Non-Primitive Data Type) Example
fruits = ["apple", "banana", "cherry", "date"]
# Accessing elements in the list
print("Fruits:", fruits)
print("Data Type:", type(fruits))
# Accessing specific elements
print("\nFirst Fruit:", fruits[0])
print("Second Fruit:", fruits[1])
# Modifying the list
fruits.append("grape")
print("\nFruits after Adding 'grape':", fruits)
# List length
print("\nNumber of Fruits:", len(fruits))
Key Differences:
Key Difference | Primitive Data Types | Non-Primitive (Reference) Data Types |
Complexity | Simple, basic values | More complex, structured data |
Memory Storage | Store the actual data | Store references to data |
Sizes | Fixed sizes | Dynamic sizes based on content |
Creation | Predefined by language | Created by the programmer |
Operations | Basic operations | Advanced operations via methods |
Examples | int, float, bool, char | lists, sets, dictionaries (Python), objects/classes (OOP languages) |
Use Cases | Basic data storage and arithmetic | Complex data structures and objects |
Conclusion
Whether you are working with simple numerical numbers or complicated data structures, knowing these data types is vital for being a competent programmer. A programmer should have a proper knowledge of data types while building some projects. Having a proper knowledge of data types helps developers in selecting the best data type according to the project requirements.
If you find this Blog helpful, then make sure to search Codewithrandom on Google for Front End Projects with Source codes and make sure to Follow the Code with Random Instagram page.