Frontend Security of a website is very important in the digital era of cyber attacks. So to protect the website from unauthorized access we need to use some advanced react tools and technology to protect our data.
The security of your web application’s front end has become more difficult and important than ever with the emergence of single-page applications (SPAs) and technologies like React.
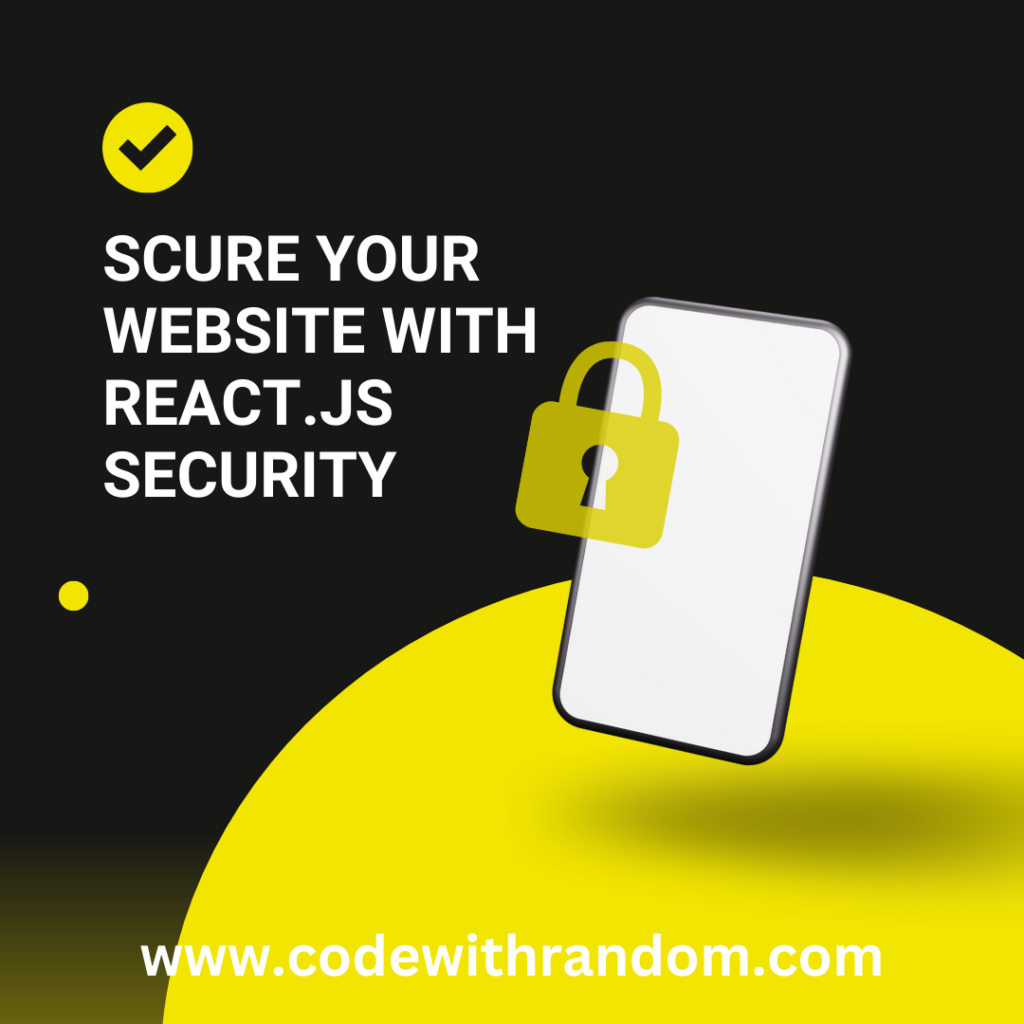
One of the most popular front-end frameworks available today is React.js, an open-source JavaScript toolkit that is scalable.
Security Attacks in React Applications
- XSS (cross-site scripting)
One important client-side vulnerability is XSS. Someone can insert harmful code into your program, which is then viewed as legitimate and run as part of the application. The app’s functioning and user data are both put at risk because of this. - Breakdown in Authentication
Insufficient or bad authorization is another frequent problem with React.js applications. As a result, attackers may hack user credentials and employ brute force methods.
Broken authorization entails a number of dangers, including the exposure of session IDs in URLs, the easy and predictable discovery of login information by attackers, the unencrypted transmission of credentials, the persistence of active sessions after logout, and other session-related elements. - Zip Slip:
The “zip slip” vulnerability, which includes taking advantage of the functionality that makes it possible to upload zip files, affects only React applications.
If the archive used to unpack the zip file is insecure, the attacker might unzip the uploaded files outside of the designated directory, giving them access to the file.
Security Methods For React.js
- Input Validation
Input validation is one of the core concepts of front-end security. Never trust data from users or outside sources. To stop malicious code from running, always validate and sanitize inputs.
import React from 'react';
import { sanitize } from 'dompurify';
function Comment({ text }) {
const sanitizedText = sanitize(text);
return <div dangerouslySetInnerHTML={{ __html: sanitizedText }} />;
}
- Preventing Cross-Site Scripting (XSS)
When an attacker inserts malicious scripts into web pages, the scripts are subsequently run by other users, leading to XSS assaults. You should sanitize and escape user-generated material to avoid XSS attacks.
import React from 'react';
import { sanitize } from 'dompurify';
function Comment({ text }) {
const sanitizedText = sanitize(text);
return <div dangerouslySetInnerHTML={{ __html: sanitizedText }} />;
}
- Content Security Policy
Set up a content security policy to limit the places from which scripts, resources, and other content can be loaded. By doing this, resource loading and unauthorized code execution are prevented.
<meta http-equiv="Content-Security-Policy" content="default-src 'self' https://api.example.com">
- Protecting API Keys
Avoid exposing sensitive data directly in your front-end code, such as API keys. Use environment variables instead, or save the data securely on the server.
// Fetching data from an API using environment variables
const API_KEY = process.env.REACT_APP_API_KEY;
fetch(`https://api.example.com/data?apiKey=${API_KEY}`)
.then((response) => response.json())
.then((data) => {
// Handle the data
});
- Implement HTTPS
Make sure your web application encrypts data while it is being transmitted over HTTPS. As a result, man-in-the-middle attacks and eavesdropping are avoided.
- Use Security Headers
To increase security, add the proper security headers to your server’s response. X-Content-Type-Options, X-Frame-Options, and X-XSS-Protection are a few important headers.
Examples (Express.js)
const express = require('express');
const app = express();
// Enable security headers
app.use((req, res, next) => {
res.setHeader('X-Content-Type-Options', 'nosniff');
res.setHeader('X-Frame-Options', 'DENY');
res.setHeader('X-XSS-Protection', '1; mode=block');
next();
});
// ... other middleware and routes
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
- Regular Updates and Dependencies:
Update all of your dependencies, including React and the libraries it uses, on a regular basis to fix any security flaws. To check for known security concerns in the dependencies of your project, use tools like npm audit. - User Authentication and Authorization:
Put strong user authentication and permission measures in place. Make sure users can only access the resources for which they have been granted access by using secure authentication protocols for user sessions, such as OAuth2 or JWT.
Live Examples of some of the security techniques
A user registration form representational a component from the React class RegisterForm. For fields like username, email, mobile number, and password, it records user inputs. The component has methods for handling input field changes (handleChange), form submission (submituserRegistrationForm), and form validation (validateForm).
It keeps the form’s state up to date, including any validation failures that are displayed beneath each input field and the user-entered data. If there are no validation problems when the user submits the form, it logs the form data to the console and displays an alert to the user indicating that the form was successfully submitted. The final step is rendering the component in the HTML root element.
Conclusion
A critical component of developing a web application is frontend security. You may drastically lower the chance of security lapses and safeguard your application as well as the data of your customers by adhering to these best practices and incorporating them into your React web app. Keeping your app up to date with the most recent security standards and procedures is important because security is a continuous process.
If you find this Blog helpful, then make sure to search Codewithrandom on Google for Front End Projects with Source codes and make sure to Follow the Code with Random Instagram page.